How to add Elements in Array in Java
Consider an array of the size n, in the given size we must add the elements in the given array.
In this tutorial we are preferred to learn how to add the elements to array in the java.
Basically, the size of the array cannot be changed in the java using static way or dynamic way. But in the java the array can be created in the dynamic way.
As per the array definition:
Array is the collection of homogenous or the similar data elements stored in a single variable name under single data type is known as Array.
Note: All the collection of elements in java will be spell it as objects or collection frame work.
In java arrays will be stored as the dynamic way under the heap area in JVM (java virtual machine). In java arrays there is no concept of storing the elements in a static way.
The main benefit of an array that can be accessed randomly but in the linked list we cannot be accessed elements directly.
Arrays in the java that are mutable data types. The size of the array in the java is fixed and, in that array, we cannot add the new elements. We have the various methods to add the elements in the array.
The following methods we used to add the elements to the array are:
- Creating the bigger array size than the current array size.
- Using the ArrayList
1. Creating the bigger array size than the current array size
For adding the elements in the java, we must create another bigger size array and copy the elements from the previous array and paste the elements into the bigger size array and place the new element to the newly created array.
We cannot add the elements directly into the
Let us see an example for adding the elements in the java array:
Example 1:
import java.util.Arrays;
public class Example
{
public static void main (String [] args)// main method
{
int array[] = {18,22,35,46,57,66};
int n = array.length; // length of the array
int myArr[] = new int[n+1];
int element = 70;
System.out.println(Arrays.toString(array));
for(int i = 0; i<n; i++)
{
myArr[i] = array[i];
}
myArr[n] = element;
System.out.println(Arrays.toString(myArr));
}
}
Output:
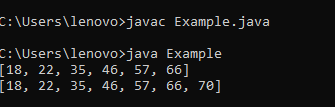
2. Using the ArrayList
As we know that the ArrayList is the collection Frame work which will be created in the dynamic way. We have change the ArrayList elements to the array using the to Array() method for adding the elements.
- Change the Array elements into ArrayList Method using asList() method for storing the elements.
- Enter the elements in to the array list for storing the elements using the add() method.
- Change the ArrayList elements into Array using the method called toArray() for storing the elements.
Let us see an example program for ArrayList for storing the elements.
Example 2:
import java.util.*;
class ArrayListDemo
{
public static void main(String args[])
{
ArrayList<String> a = new ArrayList<String>();
System.out.println("the size of the array list is =" + a.size());
System.out.println("the objects of the array list is =" + a);
a.add("APPLE");
a.add("BANANA");
System.out.println("the size of the array list is =" + a.size());
System.out.println("the objects of the array list is =" + a);
System.out.println("1-using for loop");
for (String i:a)
{
System.out.println(i);
}
System.out.println("2-using iterator");
Iterator it = a.iterator();
while(it.hasNext())
{
System.out.println(it.next());
}
a.add("MANGO");
a.add("PAPAYA");
System.out.println("the size of the array list is =" + a.size());
System.out.println("the objects of the array list is =" + a);
System.out.println("3-using list iterator forward");
ListIterator lit = a.listIterator();
while(lit.hasNext())
{
System.out.println(lit.next());
}
System.out.println("3-using list iterator backward");
while(lit.hasPrevious())
{
System.out.println(lit.previous());
}
System.out.println("the size of the array list is =" + a.size());
System.out.println("the objects of the array list is =" + a);
a.remove("APPLE");
System.out.println("the size of the array list is =" + a.size());
System.out.println("the objects of the array list is =" + a);
}
}
Output:
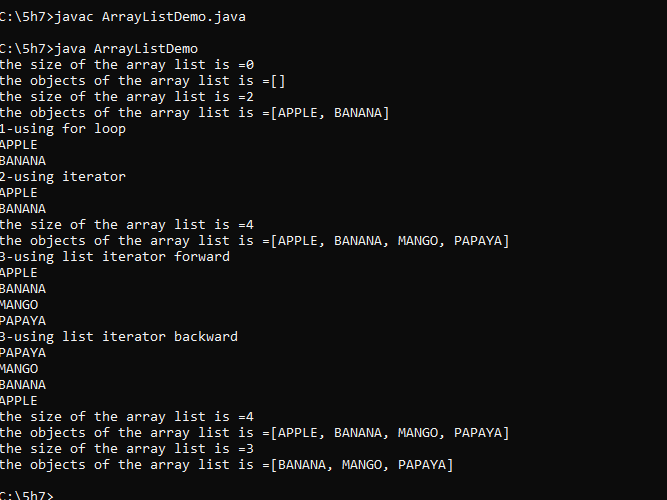