AbstractSet Class in Java
The AbstractSet class is used for the implementation of the Abstract Collection class and interface. It is the part of Collection Frameworks.
In the AbstractSet, the implementation is same as the implementing a collection by extending AbstractCollection.
The Hierarchy of the AbstractSet class.
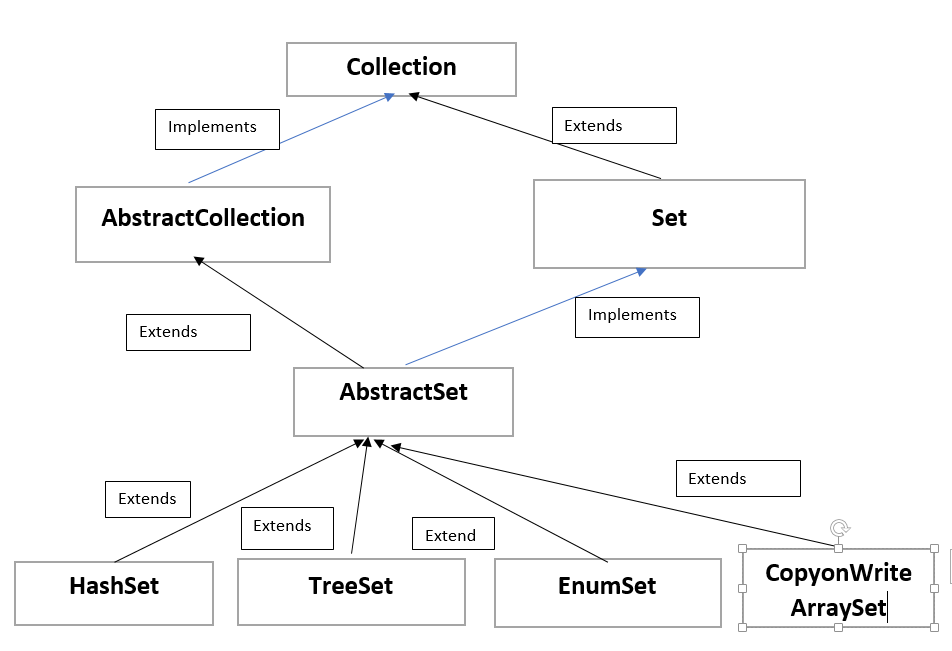
The Subclasses of AbstractSet are HashSet, TreeSet, EnumSet, CopyonWriteArraSet. The Syntax of declaring the AbstractSet is
public abstract class Abstractset<E> extends AbstractCollection<E> implements Set<E>
Constructor:
- Protected AbstractSet():
It is the default constructor of the AbstractSet with the protected access specifier. The protected access specifier doesn’t allow to create a AbstractSet object.
Methods in AbstractSet Class:
- equals(Object o):
The equals() is used to comparing the specific object with this set
- removeall():
The removeall() is used to remove all the elements from the set.
- hashcode():
The hashcode() is used to return the hash code of set.
Methods in AbstractCollection Class:
- add(E e):
The add() is used to add the specific element in the collection.
- addAll(Collection <extends E> c):
The addAll() adds all the elements from a specified collection.
- clear():
The clear() removes all the elements form the collection.
- containsAll(collection <extends E> c):
The containsAll() returns true if the collection contains the all the elements of a specified collection.
- contains(Object o):
The Conatins() returns true if the collection contains a specific element.
- isEmpty():
- The isEmpty() is used to return true if the collection doesn’t any elements.
- iterator():
The iterator method is used return iterator from the collection
- remove(Object o):
The remove() is used to remove the specific element from the collection.
- retianAll(Collection<?> c):
The retainAll() retains the elements from a specific collection.
- toArray():
The method is used to return array containing the all elements from the Collection.
- toString():
The toString() returns String representation of the Collection.
Methods of Collection interface:
- parallelStream():
The parallelStream() returns a parallel stream with the collection.
- removeIf(Predicate<? Super E> filter):
The removeIf() removes the elements from the collection if it satisfy the predicate.
- stream():
The stream() return the sequential stream of the collection.
- toArray(intFunction<T[] generator>):
The toArray() is used to return the array which contains the all the elements of the collection using the generator function.
Methods of Set interface:
- add(E e):
The add() method adds the specific element in the set if it is not already present.
- addAll(Collection <extends E> c):
The addAll() method add all the elements from a specified set if it is not already present.
- clear():
The clear() method removes all the elements form the set.
- containsAll(collection <extends E> c):
The containsAll() is used to return true if the collection contains the all the elements of a specified set.
- contains(Object o):
The contains() return true if the collection contains a specific element.
- isEmpty():
The isEmpty() is used to return true if the collection doesn’t any elements in the Set.
- iterator():
The iterator() is used return iterator from the Set.
- remove(Object o):
The remove() method removes the specific element from the Set.
- retianAll(Collection<?> c):
The retainAll() is used to retain the elements from a specific Set.
- toArray():
The toArray() is used to return array containing the all elements from the Set.
- size():
The size() method return the number of elements present in the Set.
- spliterator():
The spliterator() method create a spliterator over elements in set.
Example:
import java.io.*;
import java.util.*;
public class Main
{
public static void main(String args[])
{
try
{
/*Creating an empty treeset of int datatype using AbstractSet*/
AbstractSet<Integer> a = new TreeSet<Integer>();
/*adding elements into the tree set*/
a.add(10);
a.add(20);
a.add(30);
a.add(40);
a.add(50);
/*Printing the elemnts in the Tree set*/
System.out.println(“AbstractSet elements:”+a);
}
catch (Exception e)
{
System.out.println(e);
}
}
}
Output:
AbstractSet Elements: [10,20,30,40,50]
Example 2:
import java.io.*;
import java.util.*;
public class Main
{
public static void main(String args[])
{
try
{
/*Creating an empty treeset of int datatype using AbstractSet*/
AbstractSet<Integer> a = new TreeSet<Integer>();
/*adding elements into the tree set*/
a.add(10);
a.add(20);
a.add(30);
a.add(40);
a.add(50);
/*Printing the elemnts in the Tree set*/
System.out.println(“AbstractSet elements:”+a);
/*Creating a arraylist of int type*/
Collection<Integer> ar = new ArrayList<Integer>();
ar.add(1);
ar.add(2);
ar.add(3);
/*Printing the elements form the arraylist*/
System.out.println(“Collection Elements:”+ar);
}
catch (Exception e)
{
System.out.println(e);
}
}
}