Association in Java
In Java, association refers to the link between two classes established by their objects. One-to-one, one-to-many, and many-to-many connections are managed via association. The Association defines the multiplicity between objects in Java. It demonstrates how objects interact via communication and how they take advantage of the features and services offered by the other item. One-to-one, one-to-many, many-to-one, and many-to-many connections are all managed via association.
Examples for Association in Java
One to One
A student will have only one id which represents one to one relationship.
One to Many
A student can have multiple hobbies which represents one to many relationship.
Many to One
Students of all branches are related to one college which represents a Many to One relationship.
Many to Many
A student can access different teachers and one teacher can guide different students which represent a many-to-many relationship.
Program for Association in Java
Association.java
// Java example Program to explain the concept of Association
// Import required libraries
import java . io . * ;
import java .util . *;
// class with name branch is created
class Branch {
// instance variables of class
private String name ;
// parameterized Constructor of this class
Branch ( String name )
{
// keyword this is used to refer to the current class
this . name = name ;
}
// Method for the class
public String getBranchName ( )
{
return this . name ;
}
}
// class with name Student is created
class Student {
// instance variables of employee
private String name ;
Student ( String name )
{
// keyword this is used to refer to the current class
this . name = name ;
}
// Method of Employee class
public String getStudentName ( )
{
// returning the name of employee
return this . name ;
}
}
class Main {
// Main method
public static void main ( String [ ] args )
{
// using scanner class to read inputs from the user
// Creating object for the scanner class
Scanner sc = new Scanner ( System.in );
// Asking the user to enter the branch name
System.out.println("Enter branch name of the student ");
// Storing the the string in variable s
String s=sc.nextLine();
// Asking the user to enter name of the student
System.out.println("Enter name of the student ");
// Storing the the string in variable s2
String s2=sc.nextLine();
// Object creation for classes
Branch b = new Branch ( s ) ;
Student std = new Student ( s2 ) ;
// Accessing methods of class using objects created for the class
System . out . println ( std . getStudentName ( ) + " is student of "+ b . getBranchName ( ) ) ;
}
}
Output
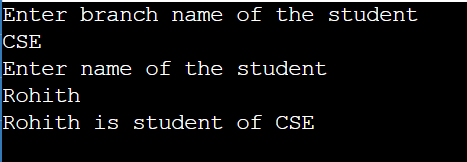
Different types of Association
- IS - A Association
- HAS - A Association
- Aggregation
- Composition
IS – A Association
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
Program for IS-A relation
IsARelation.java
// A example program to demonstrate IS – A relation
// importing necessary libraries
import java . io . *
// class with name student is created
class student{
// instance variables are created inside the class
// integer variable is created with name sal and initialized to value 40000
int sal = 40000;
}
// A class with the name IsARelation is created and using inheritance extending the properties of the parent class i.e ., student
class IsARelation extends student{
// instance variable is created with name base and initialized to value 10000
int base = 10000;
public static void main ( String args [ ] )
{
IsARelation m = new IsARelation ();
System.out.println ( " Salary is: " + m . sal ) ;
System.out.println(" Base price is: " + m . base ) ;
}
}
Output
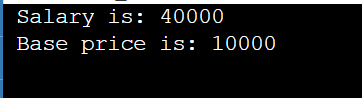
HAS – A Association
HAS – A association is further divided into two types. They are aggregation and composition.
Aggregation
The HAS-A connection is defined in Java via the Aggregation association. The one-to-one or one-way connection is followed by aggregation. When two entities are included in an aggregate composition and one of the entities has an error, the other entity is unaffected.
Aggregation.java
import java . util . * ;
import java . io . * ;
class Student
{
String name;
int year ;
String course;
Student(String name, int year, String course )
{
this . name = name ;
this . year = year ;
this . course = course ;
}
}
class Subject
{
String name ;
private List < Student > students ;
Subject ( String name , List < Student > students )
{
this . name = name ;
this . students = students ;
}
}
class College
{
String Nameofcollege ;
private List < Subject > sub ;
College(String Nameofcollege, List<Subject> sub)
{
this.Nameofcollege = Nameofcollege;
this . sub = sub ;
}
}
// main method
class Aggregation
{
public static void main ( String [ ] args )
{
Student s1 = new Student ( " Rohith ", 2002, " CSE " ) ;
Student s2 = new Student ( " Ram " , 2002, " ECE " ) ;
Student s3 = new Student ( " SAI ", 2003, " ECE " ) ;
Student s4 = new Student ( "Ravi " , 2000 , " CSE " ) ;
Student s5 = new Student ( " OM ",2002, " Mech " ) ;
//Constructing list of CSE Students.
List <Student> cse_students = new ArrayList < Student > ( ) ;
cse_students . add ( s1 ) ;
cse_students . add ( s4 ) ;
//Constructing list of ECE Students.
List < Student > ece_students = new ArrayList < Student > ( ) ;
ece_students . add ( s2 ) ;
ece_students . add ( s3 ) ;
//Constructing list of Mech Students.
List <Student> mech_students = new ArrayList < Student > ( ) ;
mech_students . add ( s5 ) ;
Subject CSE = new Subject ( " CSE " , cse_students ) ;
Subject ECE = new Subject ( " ECe " , ece_students ) ;
Subject Mech = new Subject ( " Mech ", mech_students ) ;
List < Subject > sub = new ArrayList < Subject > ( ) ;
sub . add ( CSE ) ;
sub . add ( ECE ) ;
sub . add ( Mech ) ;
// creating object of College.
College college = new College("BVRIT", sub);
System . out . print ( " Number of students of 2nd year in the college "+ college . Nameofcollege +" are 1500" ) ;
}
}
Output

Composition
Composition is a constrained version of the aggregate in which the constituents are highly interdependent. Composition, as opposed to Aggregation, represents the part-of connection. When two entities are combined, the combined object can exist without the other entity, but when two entities are combined by composition, the composed object cannot exist.
Composition.java
import java . util . * ;
class Mobiles
{
public String name ;
public String ram ;
public String rom ;
Mobiles ( String Name , String ram , String rom )
{
this . name = Name ;
this . ram = ram ;
this . rom = rom ;
}
}
class MobileShop
{
private final List < Mobiles > mobiles ;
MobileShop ( List < Mobiles > mobiles )
{
this . mobiles = mobiles ;
}
public List < Mobiles > TotalMobileInShop ( ) {
return mobiles ;
}
}
public class Composition {
public static void main ( String [ ] args )
{
Mobiles m1 = new Mobiles ( " Iphone "," 12 GB ", " 256 GB " ) ;
Mobiles m2 = new Mobiles ( " One plus 10 T pro " , " 12 GB " , " 512 GB " ) ;
Mobiles m3 = new Mobiles ( " SAMSUNG S22 ultra " , "8 GB" , " 256 GB " ) ;
List < Mobiles > m = new ArrayList < Mobiles > ( ) ;
m . add ( m1 ) ;
m . add ( m2 ) ;
m . add ( m3 ) ;
MobileShop shop = new MobileShop ( m ) ;
List < Mobiles > mob = shop . TotalMobileInShop ( ) ;
for ( Mobiles mb : mob ) {
System . out . println ( " Name : " + mb . name + " RAM : " + mb . ram + " and " + " ROM : " + mb . rom ) ;
}
}
}
Output
