ArrayList Program in Java
ArrayList Program in Java: In Java, ArrayList is a class that belongs to java.util package. It is the dynamic list that grows or shrinks at run-time as per the requirements. Because of its dynamic nature, an ArrayList is preferred over a primitive array. In other words, an ArrayList is the resizable array. The ArrayList class implements the List interface and inherits the AbstractList class.
Key Features of an ArrayList
The key features of an ArrayList are:
- Duplicate elements are allowed in the ArrayList.
- ArrayList maintains the insertion order of elements added to it.
- ArrayList is not synchronized.
- Like a primitive array, elements can be accessed randomly based on their index.
If we want to create an ArrayList, we need to create an instance of the ArrayList class.
ArrrayList<type> identifier = new ArrayList<type>();
In the above statement, identifier is the reference variable. Its name can be changed as per one’s choice. type in the angular brackets ensures what kind of array list is going to be created. For the creation of an integer list, Integer should be used inside the angular brackets. For the creation of a string list, String should be used, and so on.
FileName: ArrayListExample.java
// Importing class ArrayList import java.util.ArrayList; public class ArrayListExample { // driver method public static void main(String argvs[]) { // creating an array list of integer type ArrayList<Integer> al = new ArrayList<Integer>(); // adding elements to the list al.add(1); al.add(-9); al.add(45); al.add(443); al.add(4); // calculating the size of the list int size = al.size(); if(size > 0) { // displaying the size and the elements of the list System.out.println("Size of the array list is: " + size ); System.out.println(al ); } } }
Output:
Size of the array list is: 5 [1, -9, 45, 443, 4]
Explanation: In the code, we have created an empty list. The add() method appends an element to the list. Thus, the add() method increases the size of the list by one. Eventually, we are printing the list on the console.
Iterating an ArrayList
There are many ways to iterate over an ArrayList. A few of them are:
- Using for-loop
- Using an iterator
Let’s start with the for-loop.
Using For-Loop
Consider the following program.
FileName: ArrayListExample1.java
// Importing class ArrayList import java.util.ArrayList; public class ArrayListExample1 { // driver method public static void main(String argvs[]) { // creating an array list of integer type ArrayList<Integer> al = new ArrayList<Integer>(); // adding elements to the list al.add(1); al.add(-9); al.add(45); al.add(443); al.add(4); // calculating the size of the list int size = al.size(); System.out.println("Elements of the array list are: " ); // Iterating on every elements of the list for(int i = 0; i < size; i++) { System.out.println(al.get(i) ); } } }
Output:
Elements of the array list are: 1 -9 45 443 4
Explanation: After adding the elements to the list, we are calculating the size of the list. Then, using a Java for-loop, we are iterating over the elements of the lists. The get() method is used to access the elements of the list. It returns the element present at the given index. Thus, get(0) returns the element present at the index 0. get(1) is responsible for accessing the element present at the index 1, and so on.
Using an Iterator
Consider the following program.
FileName: ArrayListExample2.java
// Importing the class ArrayList import java.util.ArrayList; // Importing the class Iterator import java.util.Iterator; public class ArrayListExample2 { // driver method public static void main(String argvs[]) { // creating an array list of integer type ArrayList<Integer> al = new ArrayList<Integer>(); // adding elements to the list al.add(1); al.add(-9); al.add(45); al.add(443); al.add(4); // calculating the size of the list int size = al.size(); System.out.println("Elements of the array list are: " ); // Creating an iterator for the list al Iterator itr = al.iterator(); //check if the list has elements while(itr.hasNext() ) { //printing the element and move to the next System.out.println(itr.next()); } } }
Output:
Elements of the array list are: 1 -9 45 443 4
Explanation: Instead of a for-loop, we have used an iterator to iterate the elements of the ArrayList. The hasNext() method checks elements are present in the list or not. If elements are presented in the ArrayList, it returns true, else returns false. The next() method returns the next element that is getting printed on the console.
Removing Elements from an ArrayList
To remove the elements from an ArrayList, the remove() method is used. Observe the following program.
FileName: ArrayListExample3.java
// Importing the class ArrayList import java.util.ArrayList; // Importing the class Iterator import java.util.Iterator; public class ArrayListExample3 { // driver method public static void main(String argvs[]) { // creating an array list of integer type ArrayList<Integer> al = new ArrayList<Integer>(); // adding elements to the list al.add(7); al.add(8); al.add(9); al.add(17); al.add(25); // removing the element present at the index 2 al.remove(2); System.out.println("Elements of the array list are: " ); // Creating an iterator for the list al Iterator itr = al.iterator(); //check if iterator has the elements while(itr.hasNext() ) { //printing the element and move to the next System.out.println(itr.next()); } } }
Output:
Elements of the array list are: 7 8 17 25
Explanation: Before the deletion of elements, the array list looked like the following.
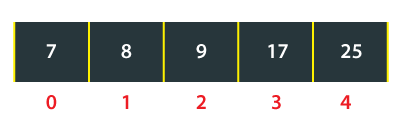
When the element at the 2nd index is deleted, the elements that followed element 9 get shifted towards the left by one place.
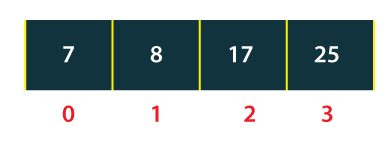
Thus, the removal of an element from an array list is a lengthy process because shifting of elements from their original position takes time.