Java IO
It is a part of java libraries but is often known as I/O streams, file I/O, and file handling. The Java I/O concept satisfies the need for input processing and output generation. It has predefined classes used for performing input-output operations for files and input-output operations performed by a user through keyboard and console. To meet the requirements above, Java employs the concept of a stream. I/O operations become faster with this concept. It enables a program to write and read entire objects to streams. One of the main applications of Java I/O is to implement the concept of file handling in Java.
File Handling
As we mentioned, stream a lot of time in the above passage, so you might be wondering what stream is and how much it aids IO operations; so let's take a look at the concept of the stream. As the name implies, it is being used to implement two functions: reading and writing data in a file. It is used to apply various tasks on a file. It does not require any different package; the java.io package consists of all file classes and allows its users to work with different file formats.
Stream
A stream is defined as the order or progression of objects that are required to support a wide range of
objects. This idea was first presented in Java 8 and is still used today. Java Stream has the following features:
- Instead of being a data structure, a stream accepts input from Collections, Arrays, and I/O Channels.
- It does not alter the original data structure; instead, they deliver the result as specified by the pipelined methods.
Before delving into the various input and output streams available in Java, let's take a look at three standard or default streams that are also the most commonly used:
- System. In: It is used to read characters entered by the user via the keyboard.
- System. Out: It is used to display the output of a program on a display device which is fulfilled by implementing the print() method.
- System.err: It displays all of the error data generated by a program on a display device like a computer screen.
With the help of the code below, we can better grasp how System.in and System.out work.
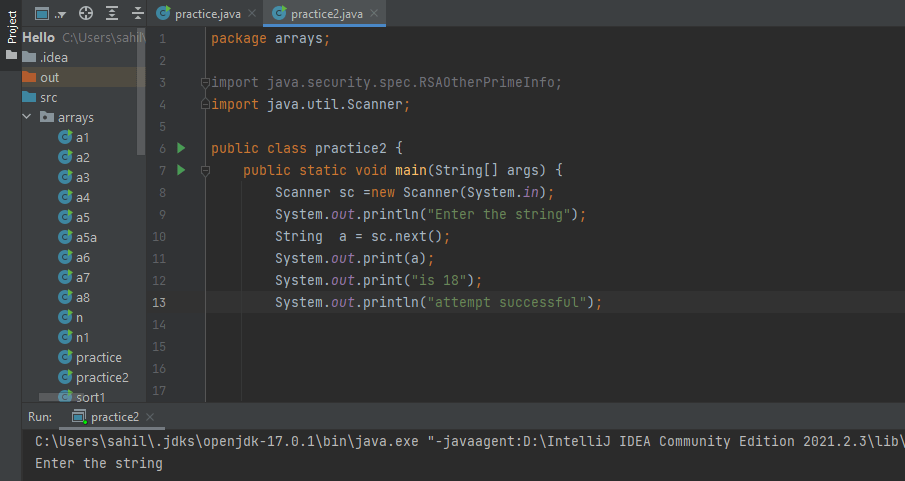
We have used the Scanner class to take input in the above code.
Scanner sc= new Scanner (System.in)
The above-highlighted line, which is used in the program at line number eight, is a Scanner class that can pass primitive types and strings using regular expressions where System.in represents the user’s keyboard.
String a = sc.next()
The above-highlighted line, which is used in the program at line number ten, takes the input from the user, which is in the configuration of String (in the above case).
We have used the print() method to print the input taken from the user and other text which we want to print. Following is the output of the code.
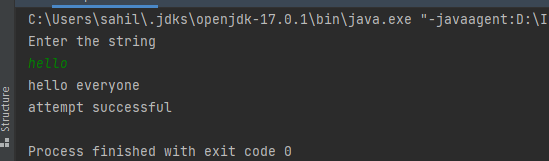
We have used two types of print: print (parameter) and println(parameter). When using the print() method, the cursor remains at the end of the text, while in the case of println, the cursor moves to the new line. Let's understand this with the following example.
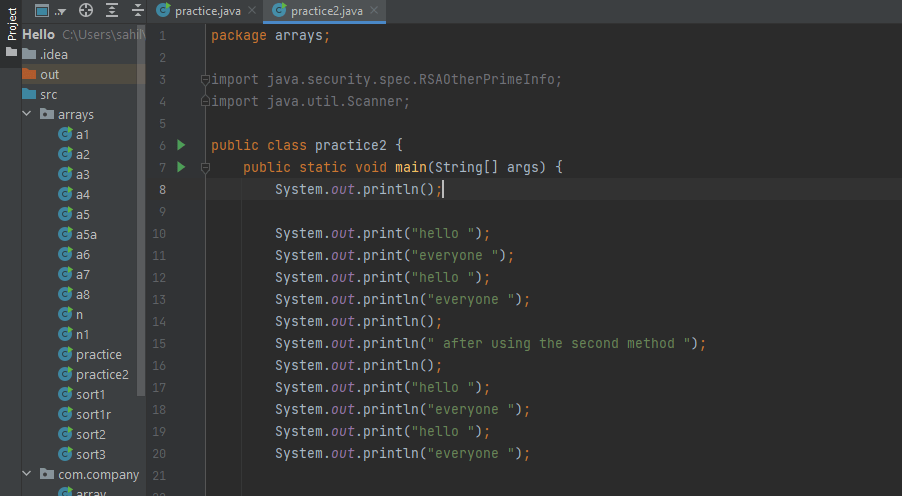
First, we printed a space so that our output looked a bit nice.
Then we have printed 4 keywords in a row.
Inline no. 13, the cursor goes to a new line and print some space, and after line no.16, the cursor again goes to a new line and print "hello everyone". To understand more precisely, let's look at the output of the above code.
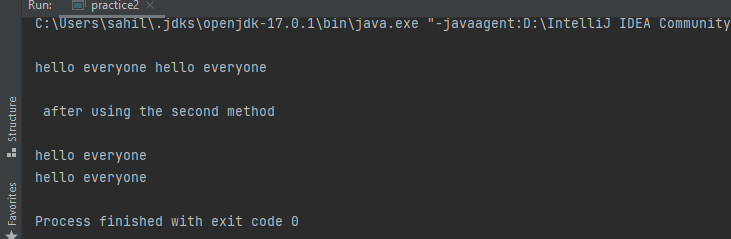
Types of Stream
Java provides two types of stream, which are explained below:
1. InputStream: Its purpose is to read data. Useful methods of InputStream are as follows.
- Public abstract int read() throws an exception: It reads the next data byte.
- Public it available() throws an exception: Its function is to estimate the byte used to read from the input stream.
- Public void close() throws an exception: It closes the present input stream as the name suggests.
2. OutputStream: Its purpose is to write data. Useful methods of OutputStream are as follows.
- Public void write(int) throws an exception: It is used to write a byte in the present output stream.
- Public void writes ( a[] )throws an exception: As the parameter suggests, it writes an array of bytes to the present output stream.
- Public void flush() throws an exception: It flushes the present output stream.
- Public void close() throws an exception: As the name suggests, the above method closes the present output stream.
Features I/O Streams
I/O stream is a powerful idea that significantly simplifies I/O operations. The java.io package consists of all the necessary classes to perform I/O operations. Let's discuss different types and features I/O streams offer a java user.
- Byte Streams: It is implemented to manage the input-output of raw binary data.
- Character Streams: It is implemented to manage the input-output of character data.
- Buffered Stream: It is used for optimizing input and output data.
- Scanning and formatting: The program can perform two functions: reading and writing.
- Data streams are implemented to manage binary input-output from primitive and String data types.
- Object Streams: As the name suggests, it manages the Input-output of objects.
Java.io Classes
The most important Java.io classes are as follows:
- BufferedInputStream
- BufferedOutputStream
- BufferedReader
- BufferedWriter
- ByteArrayInputStream
- ByteArrayOutputStream
- CharArrayReader
- CharArrayWriter
- Console
- DataInputStream –
- DataOutputStream
- File
- FileDescriptor
- FileInputStream
- FileOutputStream
- FilePermission
- FileReader and FileWriter
- FilterInputStream
- FilterOutputStream
- FilterReader
- FilterWriter
- InputStream
- InputStreamReader
- LineNumberInputStream
- LineNumberReader
- ObjectInputStream
- ObjectInputStream.GetField
- ObjectOutputStream
- ObjectOutputStream.PutField
- ObjectStreamClass
- ObjectStreamField
- OutputStream
- OutputStreamWriter
- PipedInputStream
- PipedOutputStream
- PipedReader
- PipedWriter
- PrintStream
- PrintWriter
- PushbackInputStream
- PushbackReader
- RandomAccessFile
- Reader
- SequenceInputStream
- SerializablePermission
- StreamTokenizer
- StringBufferInputStream
- StringReader
- StringWriter
- Writer
- ZipInputStream class in Java
- ZipEntry class in Java
- JarEntry class in Java
- ZipOutputStream class in Java
- Zip.InflaterInputStream class in Java
- Zip.DeflaterInputStream class in Java
- Zip.DeflaterOutputStream class in Java