Java StringReader Class
StreamReader Class technique enables character reading from a string. The java.io package contains this class. A string serves as a source in the character stream. While the Stream class is made for byte input and output, StreamReader is made for character input with a certain encoding.
Character Stream
Byte streams are frequently "wrapped" in character streams. While handling the physical I/O, the character stream makes use of the byte stream to translate between characters and bytes. The superclasses of all character stream classes are the Reader and Writer classes. The class is provided by java.io. Package. This class is used to overcome the limitations of the byte stream. The main purpose is to read characters and write the characters
Method | Description |
int read() | It is used to read a single character. |
int read(char[] cbuf, int off, int len) | It is used to read a character into a portion of an array. |
boolean ready() | It is used to tell whether the stream is ready to be read. |
boolean marksupported() | It is used to tell whether the stream support mark() operation. |
long skip(long ns) | It is used to skip the specified number of character in a stream |
void mark(int readAheadLimit) | It is used to mark the mark the present position in a stream. |
void reset() | It is used to reset the stream. |
void close() | It is used to close the stream. |
Reader class: Reader class is used to read characters from the input stream.
Declaration of StringReader class
// StringReader Creation
StringReader input = new StringReader(String data);
Methods in StringReader class
- read()
- close()
- read(char[] c, int start, int length)
- skip(longs)
- ready()
- mark()
- reset()
- mark supported()
read() method
- It is used to read single characters from the stream.
- It returns -1 if it reaches the end of the file or end of input.
- It represents whether the next character is present or not.
- Return type of read() method is int(Integer).
Syntax:
public int read()
Program:
import java.io.*;
class Example1 {
public static void main(String[] s) throws IOException {
String s1 = "JAVA";
StringReader s2=new StringReader(s1);
for(int i=0;i<s1.length();i++)
{
char c=(char) s2.read();
System . out . println(c);
}
s2.close();
}
}
Output
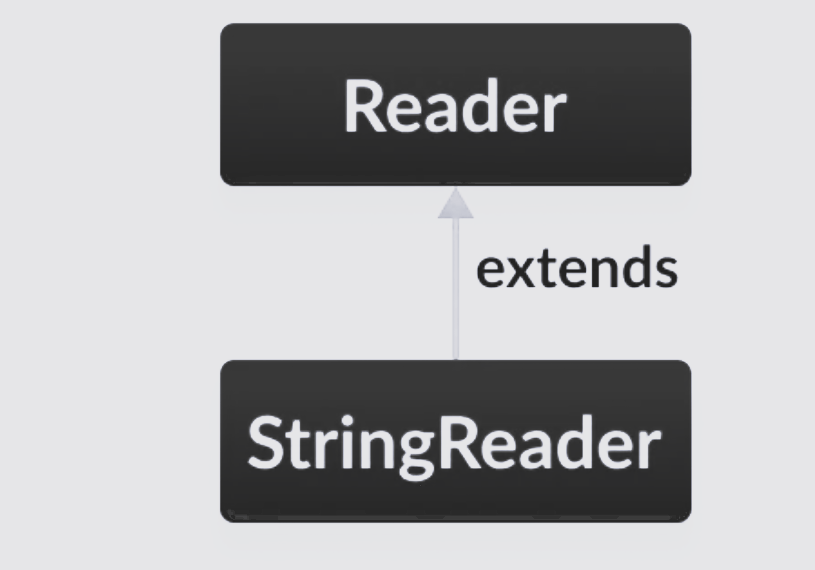
close() method
- Close method is used to close the character stream.
- If the stream has been closed, performing methods like reading (), readLine and other methods throw an IOException.
Syntax:
public void close()
Program
import java.io.*;
class Example1 {
public static void main(String[] s) throws IOException {
String s1 = "JAVA";
StringReader s2=new StringReader(s1);
for(int i=0;i<s1.length();i++)
{
char c=(char) s2.read();
System . out . println(c);
}
s2.close();
}
}
Output:

read(char[] c, int start, int length) method
- It stores the reading characters in the array form.
- It returns the count of characters.
Syntax:
public int read(char[] c, int start, int length)
c -It is the Destination buffer
start – at which index it starts writing characters.
Length -.Several characters.
Program
import java.io.*;
class Main
{
public static void main(String[] s) throws IOException
{
String s2 = "JAVAProgramming";
StringReader s3=new StringReader(s2);
char c[]=new char[10];
try
{
s3.read(c,0,5);
System.out.println(c);
}
catch (IOException E)
{
System.out.println(E);
}
}
}
Output:

skip (long s) method
- It returns the input that matches the specified pattern, ignoring delimiters.
Syntax:
public long skip(long s)
Program
import java.io.*;
class Main
{
public static void main(String s[]) throws IOException
{
String s1="JavaProgramming";
StringReader s2= new StringReader(s1);
for (int i=0;i<4;i++) {
char c =(char)s2.read();
s2.skip(3);
System.out.print(c);
}
}
}
Output:
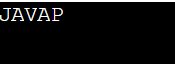
ready() method
- It checks whether the input stream is ready to be read.
- Return ready() method is boolean.
Syntax:
public boolean ready();
Program:
import java.io.*;
class Main
{
public static void main ( String s[]) throws IOException
{
String string1="Java";
StringReader string2=new StringReader(string1);
System.out.println("Print true if it is ready otherwise false:"+string2.ready());
Output:
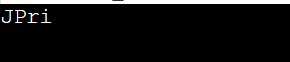
mark(int Limit)
- This method is used to represent the present position in the stream.
- Void is the return type.
Syntax: public void mark(int Limit)
Program
import java.io.*;
class Main{
public static void main(String[] s) throws IOException{
StringReader string1= new StringReader("Apple");
string1.mark(1);
System.out.println(string1.read());
string1.close();
}
}
Output:

reset () method
- It is used to reset the stream with the most recent mark.
- Return type of reset(0 method is void.
Syntax:
public void reset () throws IOException.
Program
import java.io.*;
class Main
{
public static void main(String[] s) throws IOException
{
StringReader string1 = new StringReader("Java");
string1.mark(1);
System.out.println(string1.read());
string1.reset();
string1.close();
}
Output:

mark supported () method
- It is used to check whether it supports the mark() method.
- void is the return type.
Syntax:
public void markSupported()
Program
import java.io.*;
class Main
{
public static void main(String[] s) throws IOException
{
try
{
String s1 = "JavaT";
StringReader s2= new StringReader(s1);
System.out.println("Print True if it supports otherwise False:"+ s2.markSupported());
s2.close();
}
catch(Exception e)
{
System.out.println(e);
}
}
}
Output
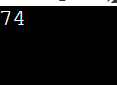