Java File Extension
A computer file's suffix is called its file extension. It is easily recognized since it appears immediately after a period (.) in the file name.
Consider the file Demo.java as an example; Demo is the file name, and .java is the file extension, both of which indicate the file format.
In this section, we will learn how to use a Java program to obtain a file's extension.
Java File Class
- The io package contains the definition of the Java File class. The java.nio package is another new Java package used to carry out input/output tasks. It first appears in JDK4.
- It is utilized in the Java program to carry out certain file operations.
- The File class has a variety of constructors and methods that can be used to rename, delete, or create new files, among other operations, using the file path name.
- A File class instance must be created to use these constructors and methods.
- Instances of the File class are immutable; thus, once they are created with a particular pathname, they cannot be modified.
How to Get the File Extension?
In Java, there are two ways to obtain file extensions.
The following program uses the File class to determine the file extension of the provided file we provide as input.
FileTypeDemo.java
import java.io.* ;
import java.nio.file.Files ;
public class FileTypeDemo
{
/*main method*/
public static void main(String ar[])
{
/* declaring a File instance with the path of the File */
File f = new File("C:/Users/vishwanth/sn/dem.txt") ; //location
/* If file exists */
if(f.exists())
{
String fType = "Undetermined" ; //undetermined
String fName = f.getName() ;
String extension = "";
int i = fName.lastIndexOf('.') ; //lastIndexOf() method
if (i > 0)
{
extension = fName.substring(i + 1) ;
}
try
{
fType= Files.probeContentType(f.toPath()) ;
}
catch (IOException ioException) //catches io exception
{
System.out.println("Cannot determine type of file "+ f.getName()+ " due to the exception: "+ ioException) ;
}
/* Print the file extension. */
System.out.println("File Extension used is: " + extension + " and is probably " + fType) ;
}
else
{
System.out.println("File does not exist!") ; //prints file does not exist
}
}
}
Output:
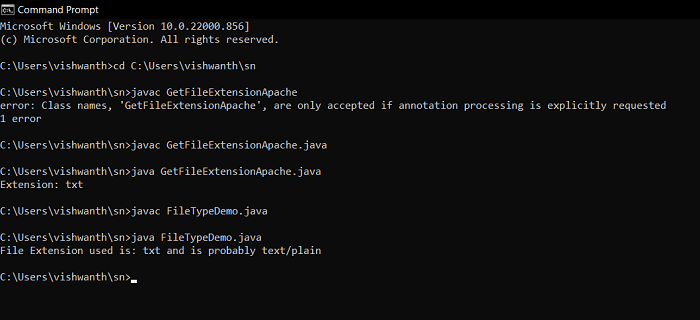
In the above-displayed image, the output for the above program is provided. As the file “dem.txt” is present, the program prints the file extension as txt for the file “dem”.
In the Java program cited above,
- It creates an instance "f" of the File class from the io package. It produces an instance of the path that was supplied to the constructor.
- The file existence is checked using an if statement on the following line. The variables for storing the file type, file name, and file extension will be created if the condition is proper.
- The last occurrence of the character supplied as an argument is returned by the lastIndexOf() function.
- substring() method is used to get the file extension after the period (.)
- The nio package's probeContentType() method returns a string indicating the content type. Due to the possibility that it will raise an IOException, it is specified inside a try-catch block.
- And last, a print() command is used to display the file extension and content type on the console.
Using Apache Commons IO Library
The Apache Commons IO component, adapted from the Java API, offers various ways to carry out multiple operations on files without first constructing an object of the file. The FilenameUtils.getExtension() method is employed in the code below to obtain the file extension.
GetFileExtensionApache.java
import java.io.IOException ;
import org.apache.commons.io.FilenameUtils ;
public class GetFileExtensionApache
{
/* Driver Code */
public static void main(String []args)
{
try
{
usingFilenameUtils();
}
catch(IOException e)
{
System.out.println(e.getMessage()) ;
}
}
public static void usingFilenameUtils() throws IOException
{
/* now, provide the file path of the required file */
String path = " C:/Users/vishwanth/sn/dem.txt" ;
System.out.println("Extension: " + FilenameUtils.getExtension(path)) ;
}
}
Output:
The getExtension() function of the FilenameUtils class is used in the Java code above to obtain the file extension without constructing a file object.
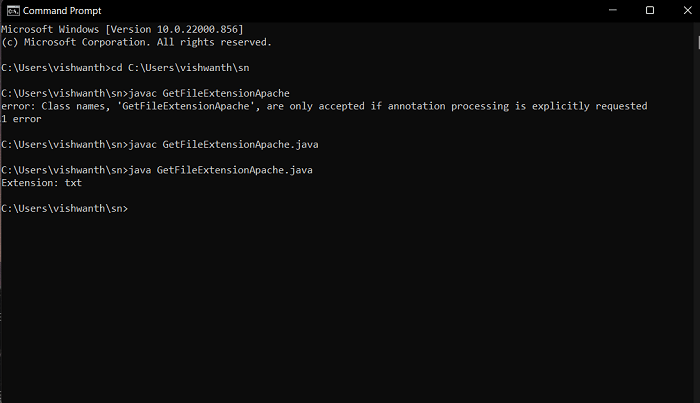
The above-displayed image shows the output for the above program. The output contains the extension of the file provided in the program. As the file “dem' is a txt file, the program displays "Extension: txt" as its output.