Java Lambda foreach
Before understanding lamda foreach, one must know about foreach loop in Java.
foreach loop in Java
Since J2SE 5.0, there has been a for-each loop in Java, also known as an extended for loop. It offers a different method for traversing an array or collection in Java. It mostly serves to iterate through an array or collection of elements. The for-each loop has the benefit of making the code more understandable and removing the risk of problems. The for-each loop is so named because it goes through each element one at a time.
The extended for loop's disadvantage is that it is unable to iterate through the elements in reverse. Since it doesn't operate on an index basis in this case, you are unable to skip any elements. Additionally, you cannot only move through the odd or even elements.
However, because it makes the code more understandable, it is advised to utilize Java for-each loop when it comes to iterating through the elements of an array or collection. The code is easier to read as a result. It takes away the chance of programming mistakes.
Syntax
The syntax of Java for-each loop consists of data_type with the variable followed by a colon (:), then array or collection.
for(data_type variable : array | collection){
//body of for-each loop
}
Filename: ForEachExample1.java
//An example of Java for-each loop
class ForEachExample1{
public static void main(String args[]){
//declaring an array
int arr[]={12,13,14,44};
//traversing the array with for-each loop
for(int i:arr){
System.out.println(i);
}
}
}
Let us see another Java for-each loop where we are going to total the elements.
Output
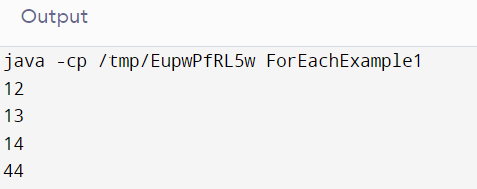
Filename: ForEachExample1.java
class ForEachExample1{
public static void main(String args[]){
int arr[]={12,13,14,44};
int total=0;
for(int i:arr){
total=total+i;
}
System.out.println("Total: "+total);
}
}
Output
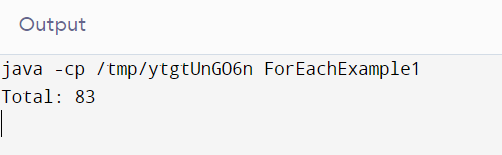
For-each loop Example: Traversing the collection elements
Filename: ForEachExample2.java
import java.util.*;
class ForEachExample2{
public static void main(String args[]){
//Creating a list of elements
ArrayList<String> list=new ArrayList<String>();
list.add("vimal");
list.add("sonoo");
list.add("ratan");
//traversing the list of elements using for-each loop
for(String s:list){
System.out.println(s);
}
}
}
Lambda Expression
In Java 8, Lambda Expressions were introduced.
A lambda expression is a brief section of code that accepts input and outputs a value. Similar to methods, lambda expressions can be used directly in a method's body and do not require a name.
Syntax
A single parameter and an expression make up the simplest lambda expression.
parameter -> expression
Put parentheses around any other parameters you want to utilize.
(parameter1, parameter2) -> expression
Use a lambda expression in the ArrayList's forEach() method to print every item in the list:
Filename:Main.java
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
numbers.forEach( (n) -> { System.out.println(n); } );
}
}
Output
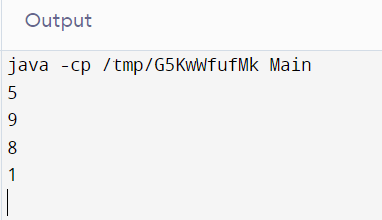
If a variable's type is an interface with only one method, lambda expressions may be placed in those variables. The return type and parameter count for the lambda expression should match those of the corresponding method. Many of these interfaces are already included in Java, such as the Consumer interface used by lists (included in the java.util package).
In order to save a lambda expression in a variable, use Java's Consumer interface:
Filename:Main.java
import java.util.ArrayList;
import java.util.function.Consumer;
public class Main {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
Consumer<Integer> method = (n) -> { System.out.println(n); };
numbers.forEach( method );
}
}
Output
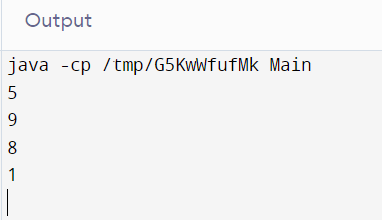
The method must contain a parameter whose type is a single-method interface in order to employ a lambda expression. The interface's method will be used to execute the lambda expression:
Filename: Main.java
Make a method that accepts a parameter that is a lambda expression:
interface StringFunction {
String run(String str);
import java.util.ArrayList;
import java.util.function.Consumer;
public class Main {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<Integer>();
numbers.add(5);
numbers.add(9);
numbers.add(8);
numbers.add(1);
Consumer<Integer> method = (n) -> { System.out.println(n); };
numbers.forEach( method );
}
}
Output
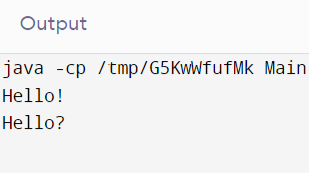
The major benefit of Java 8 functional interfaces is that we can use Lambda expressions to instantiate them and avoid using bulky anonymous class implementations.
Argument-list: It can be empty or non-empty as well.
Arrow notation/lambda notation: It is used to link arguments-list and body of expression.
Function-body: It contains expressions and statements for lambda expression.
Filename: LambdaExpressionExample.java
import java.util.ArrayList;
import java.util.List;
public class LambdaExpressionExample {
public static void main(String args[]){
List<String> list=new ArrayList<String>();
list.add("Jai");
list.add("Vivek");
list.add("Mahesh");
list.add("Vishal");
list.add("Naren");
list.add("Hemant");
list.add("Vikas");
list.add("Ajay");
list.forEach(
(name)->System.out.println(name)
);
}
}
Output
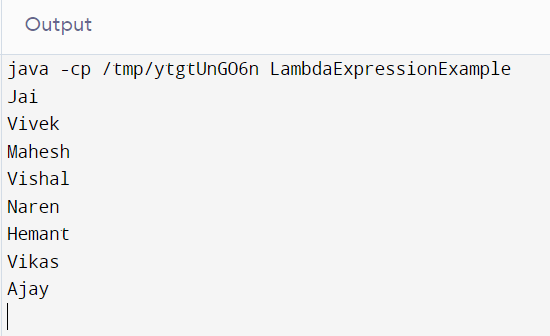