Narcissistic Number in Java
A Narcissistic number is made up of digits that have been added together and raised to powers equal to the number of digits in the original number. In those other terms, it consists of positive numbers with m digits and m th powers of digits. Other names for it include Armstrong number, Plus Perfect, and pluperfect. The sequence is A005188 from the OEIS.
Let's use instances for a better understanding of the narcissistic number.
Examples:
153 = 13+ 53 + 33 = 1 + 125 +27 = 153
8208 = 84 + 24 + 04 + 84 = 4096 + 16 + 0 + 4096 = 8208
We can see that the supplied number is a power of the entire number of digits, and the combination of the values of each digit equals the number we have considered. Consequently, the numbers provided are narcissistic.
Some of the examples of Narcissistic Numbers: 1, 2,3,4,5,6,7,8,9,150,407…
Steps Required
Following the steps required for finding the Narcissistic Number:
N can be read or initialized.
To record the specified number N's digit counting in a variable power, measure its digits.
Calculate each digit's power before adding the results in a variable total.
Comparing the variable total to N, which is provided. The provided number is narcissistic. If both numbers are equal, it is not.
Java Program for Narcissistic Number
NarcissisticExample.java
//this program is for finding the given number is Narcissistic number
//importing section
import java.util.*;
import static java.lang.Math.*;
public class NarcissisticExample
{
//function for determining the number of digits in a given number
int numDigits(int n)
{
if (n== 0)
return 0;
return 1 + numDigits(n/10);
}
//method for determining the given number is Narcissistic Number
boolean isNumber(int n)
{
// counting the digits of a given number
int power = numDigits(n);
//the given value is assigned to n
int num = n;
//result for storing the sum of the powers
int total = 0;
//the loop will iterate until the condition becomes false
while(num> 0)
{
//it will find the last digit of the given number and
//then, the power for the last digit is calculated
//after the calculation of the power, it will store in the total variable
total+= pow(num% 10, power);
//and then the last digit of the number is then removed
num= num / 10;
}
//the result(total) is then compared with the given number
return (n == total);
}
//main program
public static void main(String args[])
{
NarcissisticExample nrs = new NarcissisticExample();
Scanner sc = new Scanner(System.in);
System.out.print("Please enter the input number: ");
//reading the integer number from the user
int n= sc.nextInt();
//
if (nrs.isNumber(n))
System.out.println("The given input number "+n+" is a narcissistic number.");
else
System.out.println("The given input number "+n+" is not a narcissistic number.");
}
}
Output

This program is for finding the Narcissistic numbers of the given range.
NarcissisticExample2.java
//this program is for finding the narcissistic numbers for a given range
//import section
import java.util.LinkedList;
public class NarcissisticExample2
{
//Main section of the program
public static void main(String args[])
{
for (int i = 1; i < 1000; i++)
{
int num = i;
//a linked list was created for data storing
LinkedList<Integer> d = new LinkedList<>();
//the loop will iterate until the condition becomes false
while (num > 0)
{
//the last of the given number is added to the list
d.push( num % 10 );
//after that, the last digit is removed
num = num/ 10;
}
//the result is stored in the variable total
int total = 0;
//the loop will continue over the list
for(Integer number : d)
{
// finding the power of the digits
total += Math.pow(number, d.size());
}
//the result(total) is then compared with the given number
if(i == total)
{
//printing the range of numbers
System.out.println(i);
}
}
}
}
Output
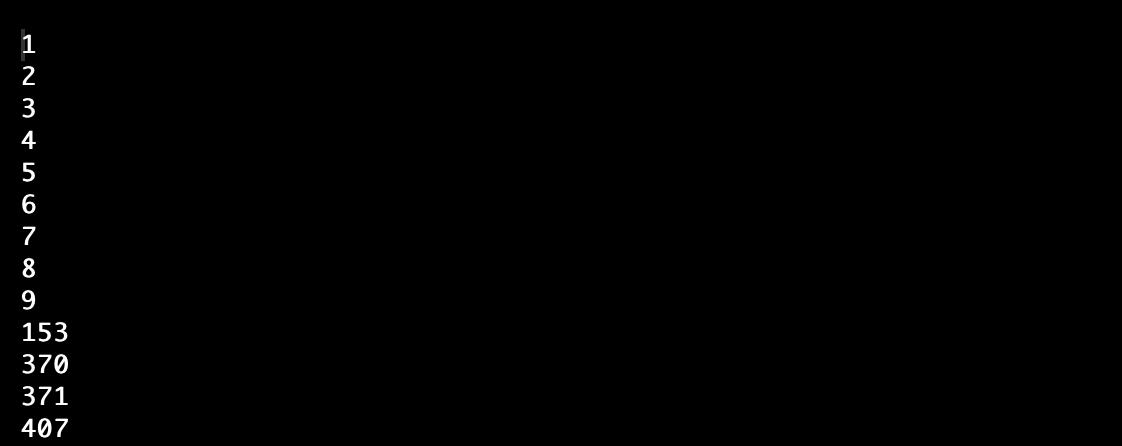