How to resolve Illegal state exceptions in Java
What is IllegalStateException?
When a method is called at the incorrect time, a runtime exception called an IllegalStateException is raised in Java. This exception is utilized to show that a method was called improperly or at the wrong time.
A thread cannot be started again once it has already been begun, for instance. If such a procedure is carried out, the IllegalStateException is raised.
Being a RuntimeException's child class, IllegalStateException is an unchecked exception. An explicit exception is thrown by the coder or the API developer when a method is invoked at the wrong time. This method is typically used to signal that a method was called in an unauthorized or inappropriate manner.
Because the IllegalStateException is an unchecked exception, it does not need to be included within the throws clause of the a method or function called Object() in native code.
Causes of the IllegalStateException
When the Java program or environment is not in a suitable state for the requested operation, the IllegalStateException is raised. This can happen when working with threads or the Java.util package's Collections framework in certain circumstances. Several scenarios where this exception may apply are shown below:
- when a thread that has already been started is given the Thread.start() function call.
- when an Iterator interface on a List is used to invoke the delete() method without first using the next() method. As a result, the List collection enters an unstable condition and raises an IllegalStateException.
- If an attempt is made to add an element to a packed queue. There will be an IllegalStateException if you add more elements than the queue can hold.
Example of an IllegalStateException
Here is an illustration of a time when the Iterator threw an IllegalMonitorStateException.
The remove() technique is used to delete an item from an ArrayList before utilising the next() method:
// ISE1.java
import java.util.ArrayList;
import java.util.Iterator;
class ISE1 {
public static void main(String args[]) {
ArrayList<String> alist = new ArrayList<String>();
alist.add("Java");
alist.add("T");
alist.add("point");
Iterator<String> i = alist.iterator();
i.remove();
}
}
Since the remove() technique is used to get rid of the previous element the iterator is referring to, the next() technique should be used before attempting to delete an element. Since the next() method has never been applied in this circumstance, the iterator tries to remove the element that comes before the first element.
Running the code above causes an IllegalStateException since the activity is unlawful:
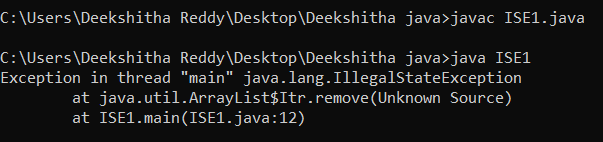
IllegalStateException Fix
It is important to make sure that no method in the code is called at an unauthorized or unsuitable time in order to avoid the Java IllegalStateException.
The problem in the example above can be resolved by utilizing the ArrayList's Iterator.next() method before calling the remove() process to remove an item from it:
ISE2.java
import java.util.ArrayList;
import java.util.Iterator;
public class ISE2 {
public static void main(String args[]) {
ArrayList<String> al = new ArrayList<String>();
al.add("Java");
al.add("T");
al.add("point");
Iterator<String> i = al.iterator();
i.next();
i.remove();
System.out.println(al);
}
}
The Iterator position is changed to the next element by calling the next() method. Removing the initial element from the ArrayList by invoking the remove() method later is a lawful action that helps resolve the exception.
Running the code above yields the desired results correctly:
