Dangling Else problem in Java
A language interpretation uncertainty is the hanging other issue. The following two types of condition executed code are both possible in programming:
1. if-then-else form
2. if-then form
When dealing with the nested if-else statement, the issue rarely arises. It is unclear which of the two if statements goes with the else clause in this conundrum. For instance, if X, then Y, then E1, else E2. There is nothing wrong with that in programming.
Grammar is used by compilers and interpreters to create the data structure needed to run programmes. A programme should, therefore, ideally have a single derivation tree. A parse tree or a derivation tree is a graphical illustration that demonstrates how production rules are used to extract strings from the grammar. There are some strings, nevertheless, that are ambiguous. The language is considered ambiguous if there are several leftmost derivations, multiple rightmost derivations, or multiple parse trees for an input text.
A grammar or string that is unclear can mean different things. In programming languages, ambiguity is frequently interpreted as a grammar mistake; this is usually unintentional.If there is more than one leftmost derivation, more than one rightmost derivation, or more than one parse tree for an input text, the language is said to be ambiguous. A grammar or string that is unclear can mean different things. In programming languages, ambiguity is frequently interpreted as a grammar mistake; this is usually unintentional.
A Fix for the Dangling Other Problem
The two methods listed below will help you avoid the "dangling else" problem:
1.Make an effort to create clear programming.
2.The brackets and indentation can be used to fix the dangling else issue.
if (condition) {
if ( 1st condition ) {
if (2nd condition ) {
}
else
{
// rest of the code
}
}
}
else
{
}
Use of if-else if-else is recommended. It provides a clear picture and knowledge of which other statement goes with which if statement.
Code for Dangling Else problem
// Importing required packages
import java.io.*;
import java . util.*;
// class with name DanglingElse
public class Main
{
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System . out . print ( " Enter the number : " ) ;
int n1 = sc . nextInt ( ) ;
if ( n1 > 0 )
{
if( n1 > 100 )
System . out . println ( " Number is more than 100 . " ) ;
}
else
{
System . out . println ( " negative number is entered " ) ;
}
}
}
Output:
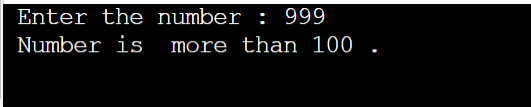
Avoiding Dangling problem by changing the syntax
Making the connection between an else and its if explicit within the syntax can help alleviate the issue. Usually, doing so helps to reduce human mistake.
- The "end if" sign, which marks the conclusion of the if statement.
- Need braces after a "if" and before a "otherwise."
- Requiring that each "if" be followed by a "else." Racket departs from Scheme by treating an if without a fallback clause as an error, thereby separating conditional expressions from conditional statements, to prevent a similar issue involving semantics rather than syntax.
- Use braces, like Swift, without exception. Python's indentation rules, which delimit all blocks, not just those in "if" statements, make this functionally true.
- The first method is to create programming languages that are clear-cut.
- Second, brackets and indentation can be used to overcome the dangling-else issues in programming languages.
The issue with Dangling Others
Initialize i=0 and j=0
if( r >= 3 )
if( r < = 10 )
i++ ;
else
j++ ;
Dangerous issues may result from dithering elsewhere. It might cause the compiler to interpret things incorrectly, which would lead to inaccurate results.
We don't know when the variable "o" will be increased in this situation. It's possible that neither the first "if" condition nor the second "if" condition will be met. Even if the first "if" condition is met, the second "if" condition could fail, which would cause the "otherwise" component to be executed. Thus, it produces unintended outcomes.
The "else" portion and the innermost "if" statement are combined in programming languages like C, C++, and Java to address the issue. On occasion, though, we like to combine the "else" clause and the outermost "if" expression.