How to Iterate a HashMap in Java?
Hash-map is a class of Java collections framework which has the functionality to implement the Map interface of Java. It stores the data in key-value pairs and can be accessed by a different type of index. The keys are unique identifiers that are associated with the values of a map. Here, one object is key, also called an index, and the other object associated with the key is called value. When we try to add the duplicate key, it will automatically replace the corresponding key data.
In this tutorial, we will understand how to iterate over a HashMap using different ways.
To use the HashMap class and its methods, we have to import the java.util.HashMap package. The HashMap class implements the Map interface.
In order to iterate a HashMap in Java, we have to understand how to create it first.
The syntax to create a HashMap is as follows:
Map<K, V> hm_name = new HashMap<>();
Here, the hashmap name is hm_name. K is the key type, and V is the type of values.
Example:
Map<Integer, String> hm = new HashMap<>();
In the above example, Integer is the type of keys, and String is the type of values.
How to iterate over a HashMap
There are various ways to iterate over a HashMap in Java. Some of them are listed below:
- Using a for loop
- Using a for-each loop
- Using the Iterator and while loop
- Using the lambda expressions
- Looping through HashMap using StreamAPI
- Using a for loop
By iterating the HashMap using the for loop, we can use the getValue() and getKey() methods. Using the getValue() and getKey() methods, key-value pairs can be iterated.
The entrySet() method of the HashMap class is used to return a set of key-value pairs of the mapped elements.
Given example explains how to iterate a hashmap using the forloop. Here, we have set.getValue() to fetch the value from set and set.getKey() to fetch the key from set.
IterateUsingForLoop.java
//importing the necessary packages import java.util.HashMap; import java.util.Map; //class to iterate the HashMap public class IterateUsingForLoop{ public static void main(String[] args) { //Creating the HashMap Map<Integer, String> hash_map = new HashMap<Integer, String>(); //Inserting elements/sets to the HashMap hash_map.put(1, "Apple"); hash_map.put(2, "Guava"); hash_map.put(3, "Banana"); hash_map.put(4, "Pineapple"); //Iterating the HashMap using for loop for (Map.Entry<Integer, String>set : hash_map.entrySet()) { //Printing all elements of a HashMap System.out.println(set.getKey() + " = " + set.getValue()); } } }
Output:
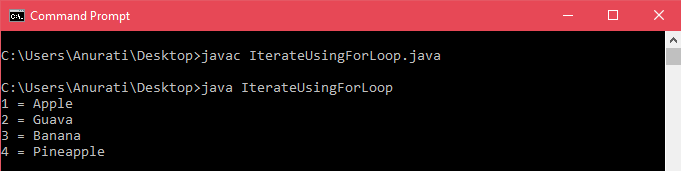
- Using a forEach loop
Here, we use the forEach loop to iterate the key-value pair of a HashMap.
Consider the following example to understand how to use a forEach loop to iterate a HashMap.
IterateUsingForEach.java
//importing the necessary packages import java.util.HashMap; import java.util.Map; //class to iterate the HashMap public class IterateUsingForEach { public static void main(String[] args) { //Creating the HashMap Map<Integer, String> hash_map = new HashMap<Integer, String>(); //Inserting elements/sets to the HashMap hash_map.put(1, "Rose"); hash_map.put(4, "Sunflower"); hash_map.put(3, "Jasmine"); hash_map.put(2, "Lily"); //Iterating the HashMap using forEach loop hash_map.forEach( (key,value) -> System.out.println(key + " = " + value) ); } }
Output:

- Using the Iterator and while loop
The Iterator is an interface used explicitly for iterating the collection elements. Here, we use the iterator() method of the Iterator interface to iterate through the key-value pairs of HashMap.
The hasNext() method returns true if the hashmap has the next element, and the next() method returns the next element of the hashmap.
Lets see the below example to understand how to use iterator() method to iterate a hashmap:
Iteration.java
//importing the necessary packages import java.util.HashMap; import java.util.Iterator; import java.util.Map; import java.util.Map.Entry; //class to iterate the HashMap public class Iteration { public static void main(String[] args) { //Creating the HashMap Map<Character, String> hash_map = new HashMap<Character, String>(); //Inserting elements/sets to the HashMap hash_map.put('A', "Arial"); hash_map.put('T', "Times New Roman"); hash_map.put('C', "Calibri"); hash_map.put('G', "Georgia"); //setting the iterator Iterator<Entry<Character, String>> it = hash_map.entrySet().iterator(); //Iterating the HashMap while (it.hasNext()) { Map.Entry<Character, String> set = (Map.Entry<Character, String>) it.next(); System.out.println(set.getKey() + " = " + set.getValue()); } } }
Output:

- Using the lambda expressions
The lambda expression is a new feature introduced in Java 8. It is a small block of code, which takes the arguments and returns a value. It is similar to a function; however, it does not require a name, and it can be implemented inside the function body.
The syntax to use lambda expression:
(argument-list) -> {body}
where,
- argument-list is the list of arguments given to the expression. It can be empty or non-empty.
- arrow-token links the argument-list and body of lambda expression.
- body contains statements and expressions for the lambda expression.
Let's consider the below example to understand how to iterate a hashmap using a lambda expression.
IterateUsingLambda.java
//importing the necessary packages import java.util.HashMap; import java.util.Map; //class to iterate the HashMap public class IterateUsingLambda { public static void main(String[] args) { //Creating the HashMap Map<Character, Integer> hash_map = new HashMap<Character, Integer>(); //Inserting elements to the HashMap hash_map.put('A', 1); hash_map.put('T', 20); hash_map.put('C', 3); hash_map.put('G', 7); //iterating hashmap using lambda expression hash_map.forEach( (k, v) -> System.out.println(k+ " = " +v) ); } }
Output:

- Looping through HashMap using StreamAPI
Iterating the HashMap using StreamAPI requires entrySet().stream() method and forEach loop. Stream API is used to process the collection of objects.
A stream is a sequence of objects with different methods that are pipelined to give the desired result. It does not modify the data structure; it just gives the result based on pipelined methods.
Here, we invoke the entrySet().stream() method which returns the stream object and the forEach loop loop iterates the elements of entrySet() method.
Consider the below example to understand how to iterate a hashmap using StreamAPI:
IterateUsingStreamAPI.java
//importing the necessary packages import java.util.HashMap; import java.util.Map; //class to iterate the HashMap public class IterateUsingStreamAPI { public static void main(String[] args) { //Creating the HashMap Map<Integer, String> hash_map = new HashMap<Integer, String>(); //Inserting elements to the HashMap hash_map.put(4, "Four"); hash_map.put(6, "Six"); hash_map.put(2, "Two"); hash_map.put(9, "Seven"); //iterating the key-value pairs in HashMap hash_map.entrySet().stream().forEach( input -> System.out.println(input.getKey() + " = " + input.getValue() ) ); } }
Output:
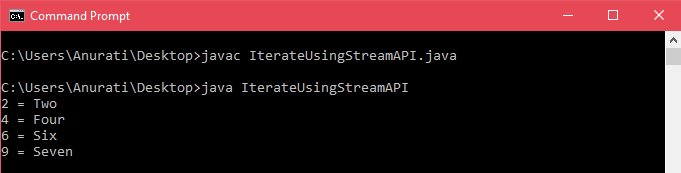
In this way, we have learned how to iterate HashMap in Java using different ways like for loop, forEach loop, an Iterator, lambda expressions, and StreamAPI.