HashMap Vs HashTable
HashMap
HashMap is the basic implementation of the map interface in Java. HashMap stores the data in key and value pairs. Keys are used to access the value of the element.
It is declared as < k ,v >.
HashMap is similar to HashTable, but HashMap is unsynchronized. It follows hashing technique to store the key and value pairs. To use HashMap, we must import the java. Util package.
Syntax
HashMap <k ,v> hashmapname = new HashMap < >( );
Here k and v represent the datatype of key and value pairs.
Example for HashMap Program
HashMap.java
// importing the required packages
// util package is required to implement hashmap
import java. util. HashMap ;
import java . io . * ;
class HashMap {
public static void main( String[] args) {
// create a hashmap
HashMap < String , Integer> h1 = new HashMap < > ();
// adding elements to hashmap
h1 . put ( " Hi " , 2);
h1 . put ( " Hello " , 3);
h1 . put ( " Everyone " , 8);
System . out. println ( " HashMap is : " + h1);
} // Main
}// HashMap
Output

Operations on HashMap
- Add elements
- Remove elements
- Access elements
- Alter elements
Adding Elements to HashMap
To add elements to HashMap, we use the put ( ) method.
AddElements.java
import java. util. HashMap;
import java . io . * ;
class AddElements {
public static void main( String[] args) {
// Object creation for hashmap with name h
HashMap < String, Integer> h = new HashMap < > ();
// add elements to hashmap
h . put (" One " , 1);
h . put (" Two " , 2);
h . put (" Three " , 3);
System . out. println("HashMap is : " + h);
} // Main
} // AddElements
Remove Elements in HashMap
To remove elements in HashMap, we use the remove ( ) method.
RemoveElements.java
import java. util. HashMap;
import java . io . * ;
class RemoveElements {
public static void main( String[] args) {
// create a hashmap
HashMap < String, Integer> h2 = new HashMap < > ();
// add elements to hashmap
h2 . put ( " One " , 1);
h2 . put ( " Two " , 2);
h2 . put ( " Three " , 3);
h2 . put ( " Four " , 4);
System . out. println ( " Before removing elements the HashMap is : " + h2 );
// removing the element with the key "Two."
h2 . remove ( " Two " ) ;
System . out. println ( " After removing element the HashMap is : " + h2 ) ;
} // main
} // RemoveElements
Output

Access Elements in HashMap
To access the elements in HashMap, we use the get method
AccessElement.java
import java. util. HashMap;
import java . io . * ;
class AccessElement {
public static void main( String[] args) {
// create a hashmap
HashMap < Integer,String> h3 = new HashMap <> ();
// add elements to hashmap
h3 . put(1," One ");
h3 . put(2," Two ");
h3 . put(3," Three ");
h3 . put(4," Four ");
// Printing the HashMap elements
System . out. println (" Elements of the HashMap is : " + h3);
// Accessing the elements of the HashMap
String value = h3 . get (3);
// Printing the value of element at index 3
System . out . println (" Value at index : " + value);
} // main
}
Output

Change Elements in HashMap
HashMap is mutable. We can change the data of the HashMap by using replace ( ) method.
AlterElements.java
import java. util. HashMap;
import java . io . * ;
class Alterelements {
public static void main( String[] args) {
// create a hashmap
HashMap < Integer,String> h4 = new HashMap <> ();
// add elements to hashmap
h4 . put(1," One ");
h4 . put(2," Two ");
h4 . put(3," Three ");
h4 . put(4," Four ");
// Printing the HashMap elements
System . out. println (" Elements of the HashMap before changing the data is: " + h4);
// Changing the value of element at index 4 from four to changed
h4. replace (4," changed ");
// Printing the Elements of HashMap after altering it
System . out. println (" Elements of the HashMap after changing the data is : " + h4);
} // Main
} //AlterElements
Output

HashTable
HashTable is the basic implementation of the map interface in Java. HashTable stores the data in key and value pairs. Keys are used to access the value of the element.
It is declared as < k ,v >. It is a synchronised data structure.
Syntax
HashTable <k ,v> hashtablename = new HashTable < >( );
Example for HashTable Program
HashTableExample.java
import java.util.*;
class HashTableExample{
public static void main(String args[]){
// Hashtable declaration
Hashtable < String, Integer > h1 = new Hashtable < String ,Integer>();
// Adding data to Hashtable
h1 . put ( "Om" , 201 ) ;
h1 . put ( "Sai" , 202 ) ;
h1 . put ( "Ram", 203 ) ;
// Accessing the data of Hashtable
for( Map . Entry r : h1 . entrySet ( ) ){
System . out . println ( r . getKey ( ) + " " + r . getValue ( ) ) ;
} // for
} // main
} // HashTableExample
Output
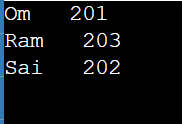
Operations on HashMap
- Add elements
- Remove elements
- Access elements
Add Elements to HashTable in Java
To add elements to HashTable, we use the put ( ) method.
AddElements.java
import java .io .*;
import java . util . *;
class AddElements {
public static void main ( String args [ ] ) {
// Hashtable declaration
Hashtable < String, Integer > h1 = new Hashtable < String ,Integer>();
// Adding data to Hashtable
h1 . put ( "Om" , 201 ) ;
h1 . put ( "Sai" , 202 ) ;
h1 . put ( "Ram", 203 ) ;
// Printing the data of Hashtable
System . out . println (h1) ;
} // main
} // AddElements
Output

Remove Elements to HashTable in Java
To add elements to HashTable, we use the remove ( ) method.
RemoveElements.java
import java .io .*;
import java . util . *;
class RemoveElements {
public static void main ( String args [ ] ) {
// Hashtable declaration
Hashtable < String, Integer > h1 = new Hashtable < String ,Integer>();
// Adding data to Hashtable
h1 . put ( "Om" , 201 ) ;
h1 . put ( "Sai" , 202 ) ;
h1 . put ( "Ram", 203 ) ;
// Before removing the element from Hashtable
System . out . println ("Hashtable before removing is"+h1) ;
// Printing the data of Hashtable
h1 .remove("Om");
System . out . println ("After removing element from Hashtable"+h1) ;
} // main
} // RemoveElements
Output

Access Elements of HashTable in Java
AccessElements.java
import java.util.*;
class AccessElements{
public static void main(String args[]){
// Hashtable declaration
Hashtable < String, Integer > h1 = new Hashtable < String ,Integer>();
// Adding data to Hashtable
h1 . put ( "Om" , 201 ) ;
h1 . put ( "Sai" , 202 ) ;
h1 . put ( "Ram", 203 ) ;
// Accessing the data of Hashtable
for( Map . Entry r : h1 . entrySet ( ) ){
System . out . println ( r . getKey ( ) + " " + r . getValue ( ) ) ;
} // for
} // main
} // AccessElemets
Output
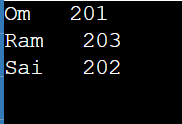
Differences between HashMap and HashTable
HashMap | HashTable |
HashMap does not have synchronisation. Without suitable synchronisation code, it cannot be shared by several threads and is not thread safe. | The HashTable is synchronised. It is shared across numerous threads and is thread-safe. |
Because multiple threads can run simultaneously, the HashMap object is not thread-safe. | The object of the HashTable may only be operated by one thread at a time. It is, therefore, thread-safe. |
HashMap supports a single null key as well as multiple null values. | Invalid keys and values are not permitted in HashTables. |
The lack of waiting time for threads results in high performance. | Low-performance results from an increase in the thread's waiting time. |
By calling this function, we can make the HashMap synchronised. Map m = Collections.synchronizedMap(hashMap); | Internal synchronisation of the HashTable prevents unsynchronization from occurring. |
Iterator iterates over HashMap. | Enumerator and Iterator iterate through the HashTable. |
HashMap derives from the AbstractMap class. | The Dictionary class is inherited by HashTable. |
The HashMap iterator is reliable. | The iterator in a HashTable is not error-proof. |
It has no legacy. | The legacy is there. |