Duodecimal in Java
Duodecimal is a notation style in which a number with a base of 12 is referred to be a duodecimal number. In Java, we can use to convert duodecimal integers to their appropriate binary, octal, decimal, hexadecimal, or other base numbers. To do the following conversion in Java, we can utilize existing packages or user-defined methods.
Steps to find Duodecimal Number:
To find the duodecimal number of any given number, perform the following steps:
- As input, take a hexadecimal number from the user.
- Create a custom method to convert it to decimal.
- We also write a user-defined function that converts decimal numbers to duodecimal numbers.
- Return the duodecimal number.
DuoDecimalDemoExample.java
import java.util.*;
class DuoDecimalDemoExample {
// To discover the equivalent duodecimal number of a given hexadecimal number , define the class DuodecimalDemoExample.
public static String convToDeci (String val , int bas , Map<Character , Integer> hexToDec)
// construct a convToDeci () method that returns the decimal equivalent of the given hexadecimal value
{
int add = 0;
int pow = 0;
String tem_Data = val;
// finding corresponding decimal number
for (int i = tem_Data.length () - 1; i >= 0; i--) {
int value = tem_Data.charAt (i) - '0';
if (bas == 16 && hexToDec.containsKey (tem_Data.charAt (i))) {
value = hexToDec.get (tem_Data.charAt (i));
}
add += (value) * (Math.pow (bas, pow++));
}
return String.valueOf (add);
}
public static String convertToDuoDecimal (String val , int bas , Map<Integer , Character>dectoHex , Map<Character , Integer>hextoDec)
// develop a convertToDuoDecimal () method that converts a decimal number to a Duodecimal value and returns it to the main () method
{
String val1 = val;
int nBase = bas;
// verify base is decimal or not
if (nBase != 10) {
// If the base is not 10, it calls the convToDeci () function, which returns the appropriate decimal number of the provided number.
val = convToDeci (val, bas, hextoDec);
// We update the value of newBase to 10 after converting the number.
nBase = 10;
}
int tempo = Integer.parseInt (val1);
int rem;
String duoDecimal = "";
char duoChars [] = {'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B'};
while (tempo > 0)
{
rem = tempo % 12;
duoDecimal = duoChars [rem]+duoDecimal;
tempo = tempo / 12;
}
return duoDecimal;
}
public static void main (String [] args)
{
String val1;
Scanner sc0 = new Scanner (System.in);
System.out.print ("enter hexadecimal number: ");
val1 = sc0.nextLine ();
sc0.close ();
Map <Character , Integer> hexToDec = new HashMap<> ();
Map <Integer , Character> dectoHex = new HashMap<> ();
for (int i = 0; i < 6; i++) {
dectoHex.put (10 + i , (char) ('A' + i));
hexToDec.put ((char) ('A' + i) , 10 + i);
}
System.out.println ("Duodecimal : " + convertToDuoDecimal (val1 , 16 , dectoHex , hexToDec));
}
}
Output:
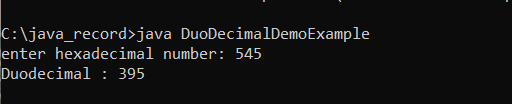
Other than hexadecimal, we must construct user-defined methods such as the convertToDec () function, which will convert the supplied integer into decimal and return it for calculating the duodecimal number.