Character Array in Java
A character array is an array which holds values of character data types. It is different from a string array. The character array, string, and StringBuffer classes in the Java programming language all support the UTF-16 format. As arrays are immutable in Java, with the help of character arrays, we can manipulate the arrays. It is very efficient and faster in execution.
Characters in Java
Char is a data type which is used to hold values like
- Alphabets
- Special Characters (@,# , $,%)
- Numbers between (0 to 65535)
- Unicode characters
Char data type is used to declare character types of variables and methods. Its default size is 2 bytes.
The syntax for Declaration of Character arrays
To declare character arrays, we use the char keyword and two square brackets [].
Syntax:
char array name [];
We can also place the square brackets before the array name.
(OR)
char [] array name;
The syntax for initialization of Character arrays
Syntax:
char array name [] = new char [size of the array];
Example for initialization of character array
Main.java
public class Main
{
public static void main(String[] args) {
// Declaration character array
char a[]=new char[6];
//Initializing the values to a character array
a[0]='r';
a[1]='0';
a[2]='h';
a[3]='i';
a[4]='t';
a[5]='h';
// Printing the values of the character array
System.out.println(a[0]);
}
}
Explanation
In the above program, a character array with the name 'a' is declared and initialized with characters of the word "Rohith". We will print the characters using the println method.
Loops for Iterating the character arrays
To iterate the character arrays, we use loops like for, while, do while and for each loop in Java.
Iterating using for each loop
Program for iteration of character arrays
Main.java
import java.util.*;
public class Main
{
public static void main(String[] args) {
char a[]=new char[6];
a[0]='r';
a[1]='0';
a[2]='h';
a[3]='i';
a[4]='t';
a[5]='h';
// printing character array values using for each loop
for(char c:a) {
System.out.println(c);
}
}
}
Output
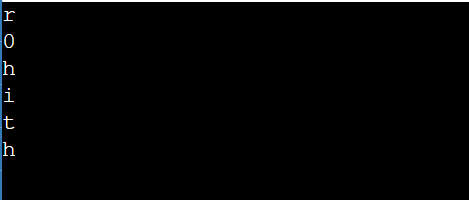
Explanation
In the above program, a character array with the name 'a' is declared and initialized with characters of the word "Rohith". We will print the characters using each loop method.
Iterating using for loop
Program for iteration of character arrays
Main.java
public class Main {
public static void main(String[] args) {
char[] A = {'H', 'e', 'l', 'l', 'o'};
// printing character array values using for loop
for (int i=0; i<A.length; i++) {
System.out.println(A[i]);
}
}
}
Output
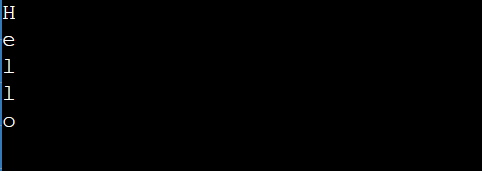
Explanation
In the above program, a character array with the name 'a' is declared and initialized with characters of the word "Hello". We will print the characters using for loop method.
Sorting a character array
To sort a character array, we will use Arrays.sort() method
The syntax for sorting Character arrays
Syntax:
Arrays.sort(ArrayName) ;
Program for sorting character arrays
Main.java
import java.util.Arrays;
public class Main {
public static void main(String[] args)
{
// Declaring a character array with random characters
char[] A = {'v', 'a', 'c', 'g', 'r','q'};
// Sorting the array using Arrays.sort() method
Arrays.sort(A);
// By default, the sorted array will be in ascending order.
System.out.println("Sorted array is");
System.out.println(Arrays.toString(A));
}
}
Output

Explanation
In the above program, a character array with the name 'a' is declared and initialized with characters. By using Arrays. sort(), we will sort the characters in ascending order by default.
Length of a character array
To find the length of a character array, we use Arrayname. Length method.
Syntax:
Array name.length;
Program for finding the length of character arrays
Main.java
public class Main
{
public static void main(String[] args)
{
// Declaring the array and initializing it with characters.
char A []= {'R', 'o', 'h', 'i', 't','h'};
// Using the length method to find the length of an array.
System.out.println("length of the character array is "+A.length);
}
}
Output

Explanation
In the above program, a character array with the name 'a' is declared and initialized with characters of the word "Rohith". We will use the length method to find the length of a character array.
To convert a string array into a character array.
The toCharArray() function makes it simple to change a string array into a character array. Changing a string field into a character field is the simplest.
Program to convert string arrays into character arrays
Main.java
class Main
{
public static void main(String[] args)
{
String s = "Hello";
char[] a = s.toCharArray();
for(char c : a)
{
System.out.println(c);
}
}
}
Output
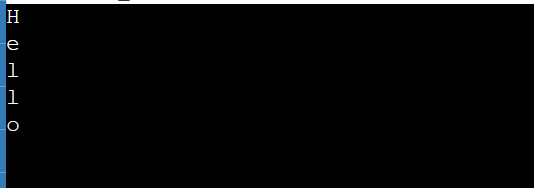