Checked vs Unchecked Exceptions in Java
In this tutorial, we will discuss Java's checked and unchecked Exceptions.
Exception
An exception is an undesirable event that disrupts the normal flow of the program. An exception is thrown at runtime.
It indicates some unusual event. Usually, it might be an error. In Java, an exception is dealt with by utilizing the following keywords
- try
- catch
- throw
- throws
- finally
- RuntimeException: a particular case of this kind addresses a programming error, and ordinarily, we shouldn't throw and catch runtime exemptions. NullPointerException is a very notable runtime particular case in Java. Up to this point, we have Error and Exception that may be RuntimeException or compile time and then addresses three types of errors and exceptions in JDK. Under the errors and exceptions, there are a ton of subclasses. For instance, the IOException addresses an overall I/O mistake and subclass. FileNotFoundException is a more specific case showing a record can't be situated on the circle. That is the way Java exemption API is coordinated.
There are two types of exceptions involved in a java programming language:
- Checked exceptions
- Unchecked exceptions
Checked Exceptions
Checked exceptions are checked at compile time of the program. In a program, if some part of the code throws an error or exception, it must throw it to the console by using the throws keyword or handle the exception handling keywords.
Checked exceptions are further classified into two types:
- Fully checked exceptions:
A fully checked exception is reviewed by child classes, like IOException, and InterruptedException.
- Partially checked exceptions:
A partially checked exception is an exception in which the few child classes are unchecked like an exception.
These exceptions are categorized as checked exceptions and grouped by package.
The following are the few exceptions involved or defined in the Java.lang package:
- I/O Exception
- ClassnotFound Exception
- Socket Exception
- SQL Exception
- CloneNotSupportedException
- InterruptedException
Similarly, we will get more checked exceptions in java.net, java.SQL, etc. packages.
Here is an example program of Checked exception. Initially, we will check whether we are getting an exception and then try to handle that exception.
For instance, consider the Java program that opens the file from the local storage which has the “C:\\Users\\malip\\jp\\test.txt” path and prints the first two lines of it. The below program doesn't compile since the main method uses FIleReader and throws a FileNotFoundException, one of the checked exceptions. But again, it throws two more exceptions of IOException because of readLine and close methods.
Code:
import java.io.*;
class Tutorialandexample {
// Main method
public static void main(String s[])
{
// Reading file from the local directory
FileReader f = new FileReader("C:\\Users\\malip\\jp\\test.txt");
BufferedReader br = new BufferedReader(f);
// Printing the first two lines of the file
for (int counter = 0; counter < 2; counter++)
System.out.println(br.readLine());
// Closing file
br.close();
}
}
Input:
test.txt
Welcome to Tutorialandexample.
Today, we will discuss checked exceptions.
Output:
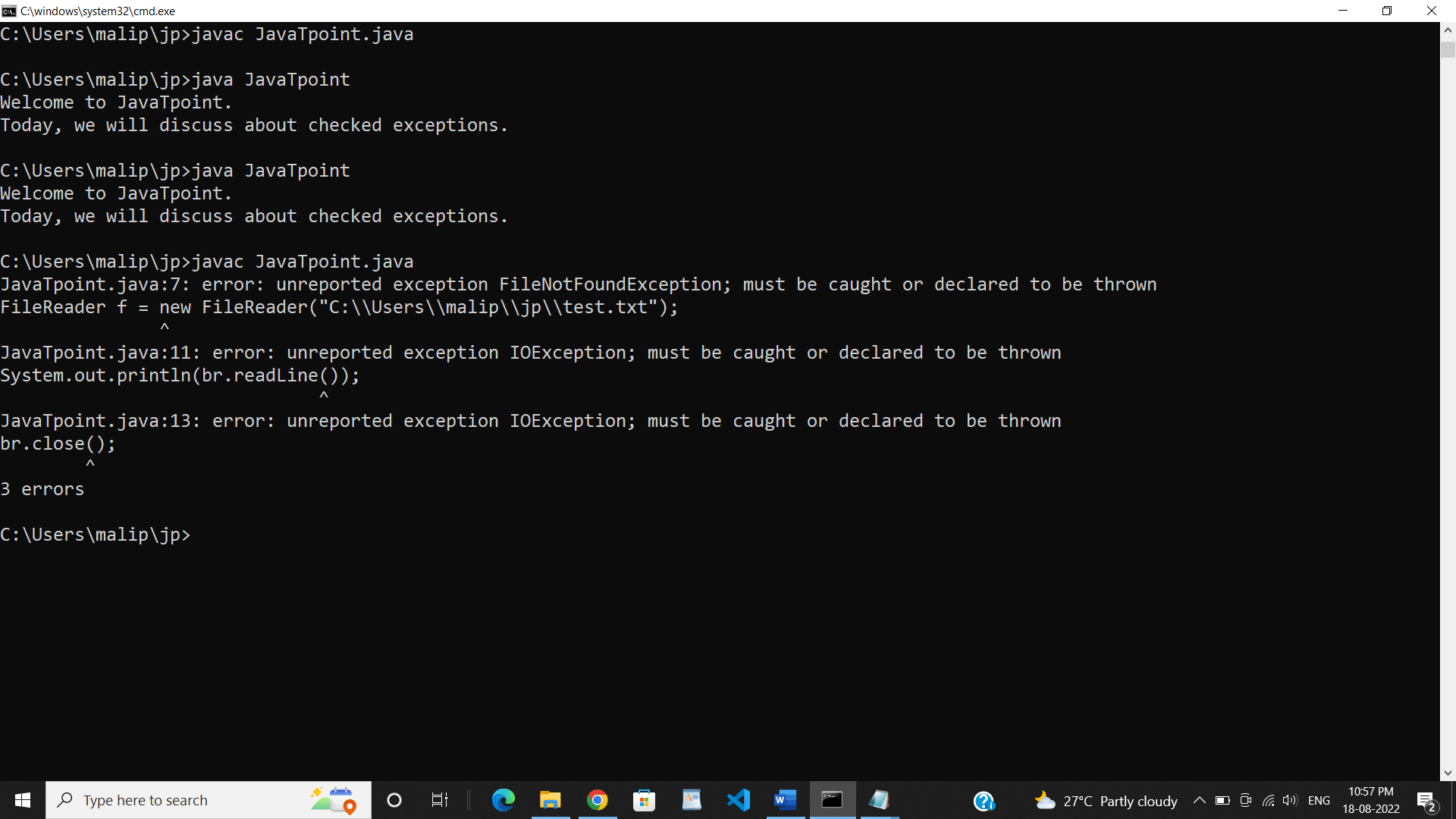
To resolve the above program, we either need to specify the throws block or must handle it by try and catch block. In the below program, we have used throws to handle the exception. Generally, the obtained exception is a FileNotFoundException. Still, as it is a subclass of IOException, we can declare IOException in the throws list to make the above program compiler-error-free.
Program:
// Java program to Handle FileNotFoundException
import java.io.*;
class Tutorialandexample {
// Main method
public static void main(String s[]) throws IOException
{
// Reading file from the local directory
FileReader f = new FileReader("C:\\Users\\malip\\jp\\test.txt");
BufferedReader br = new BufferedReader(f);
// Printing the first two lines of the file
for (int counter = 0; counter < 2; counter++)
System.out.println(br.readLine());
// Closing file
br.close();
}
}
Input:
test.txt
Welcome to Tutorialandexample.
Today, we will discuss checked exceptions.
Output:
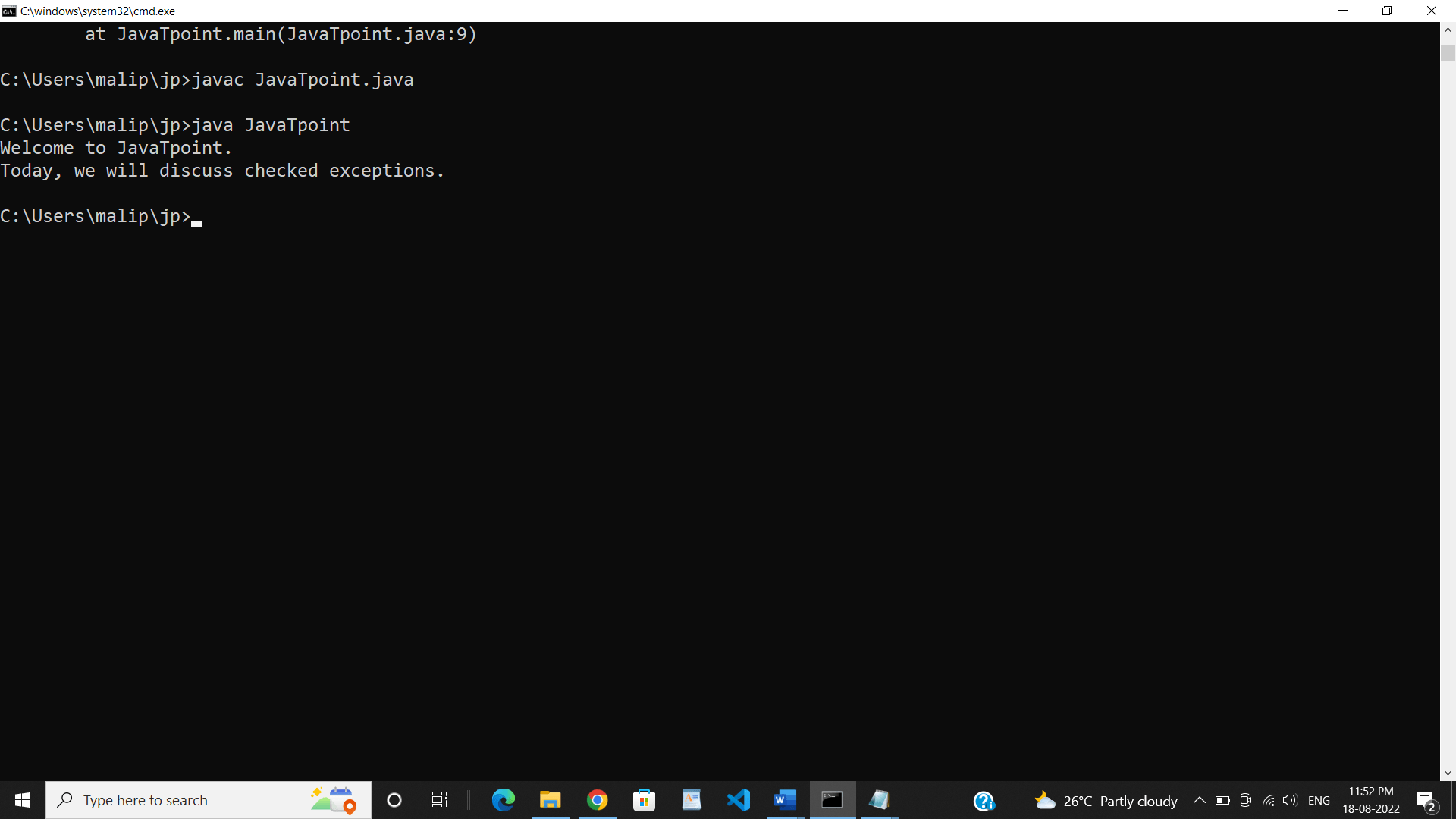
Unchecked Exceptions
Unchecked exceptions are the exceptions that are not checked at compile time. In compiled languages, all acquired exceptions are unchecked, so it is not mandatory for the compiler to handle or specify the exception. It is based on the programmers developing and determining or catching exceptions.
These exceptions are categorized based on the packages. The below mentioned are standard unchecked exceptions in Java.
- ArithmeticException
- NumberFormatException
- IndexOutOfBoundsException
- ArrayIndexOutOfBoundsException
- StringIndexOutOfBoundsException
- NegativeArraySizeException
- NullPointerException
- SecurityException
Similarly, we will get more Unchecked exceptions in java.net, java.sql, etc. packages.
Program:
// Java Program for Un-checked Exceptions
class Tutorialandexample
{
// Main method
public static void main(String s[])
{
int x = 0;
int y = 10;
int z = y / x;
System.out.println(z);
}
}
Output:
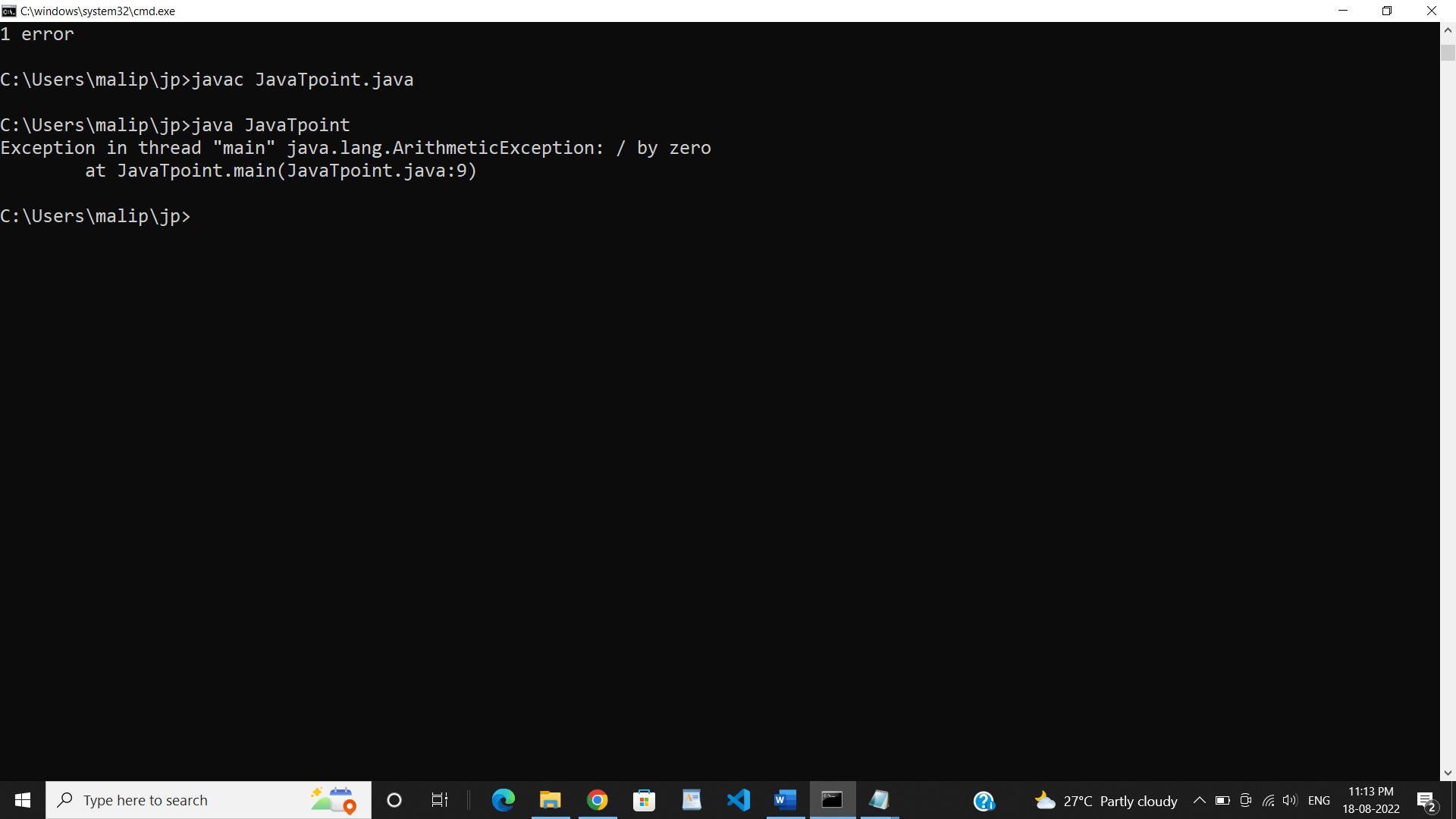
In the above output, we can see that the program is successfully compiled, but at the run time, we got an ArithmeticException, one of the Unchecked exception types.