How to sort an array in Java
Sorting is the process of arranging the elements of a list or array in a specific order, either ascending or descending. The sorting criterion numerical and alphabetical is commonly used to sort.
There are various sorting techniques to sort an array using loops. However, Java provides two in-built methods to facilitate the sorting of array elements.
In this tutorial, we will learn how to sort an array using the following methods:
- Using Arrays.sort() Method
- Using reverseOrder() Method
- Using Java for loop
Using Arrays.sort() Method
The Arrays.sort() method sorts the elements of primitive type and objects used to implement the comparable interface.
The Arrays.sort()method usesthe Dual Pivot Quicksort algorithm to sort primitive type elements and iterative mergesort to sort the objects.However, in the latest versions of Java, it uses Timsort.And its complexity is O(n log(n)).
The main task of Arrays.sort() is to parse the array given in the argument. It can also be used to sort the sub-arrays. It is a static method and does not return anything. It can sort the arrays of type integer, float, double, char.
Syntax:
sort(array_name);
The syntax to sort the selective array / subarray is:
sort(array_name, fromIndex, toIndex);
where,
fromIndex is the start of the subarray (inclusive), and toIndex is the end of the subarray (exclusive).
Let’s see some examples to sort arrays of different primitive types:
- Sorting a Numeric array:
To sort an integer array in ascendingorder, we directly use the Arrays.sort(). It sorts the arrays in ascending order by default. We can also use the toString() method of the Arrays class to print the array.
Note: We can sort double, float, long arrays just like the integer array using sort() method.
NumericArraySort.java
//importing necessary libraries import java.util.Arrays; public class NumericArraySort { public static void main(String[] args) { //defining an integer array int[] array_name = {45, 21, 66, 87, 32, 77, 1, 8, 33}; System.out.printf("Array before sorting: %s", Arrays.toString(array_name)); //sorting the array in ascending order Arrays.sort(array_name); System.out.printf("\n\nArray after sorting: %s\n", Arrays.toString(array_name)); } }
Output:
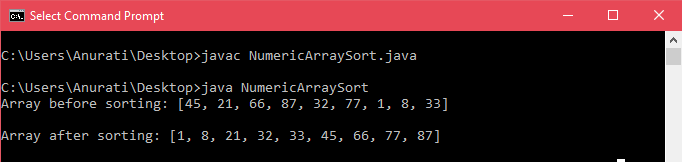
To sort an array in descending order the sort() method uses a second parameter Collections.reverseOrder(). Now we will see an example to sort an integer array in descending order.
Note: TheCollections.reverseOrder()is not applicable for primitive types. It works fine with arrays of objects.
NumericArrayRevSort.java
//importing necessary libraries import java.util.Arrays; import java.util.Collections; public class NumericArrayRevSort { public static void main(String[] args) { Integer[] array_name = {45, 21, 66, 87, 32, 77, 1, 8, 33}; //Printing the unsorted array System.out.printf("Array before sorting: %s", Arrays.toString(array_name)); //sorting array in descending order Arrays.sort(array_name, Collections.reverseOrder()); //Printing sorted array System.out.printf("\n\nArray after sorting in descending order: %s\n", Arrays.toString(array_name)); } }
Output:

- Sorting a String array:
Like an integer array, we can sort string array using the sort() method of Array. When the string array is passed to the sort() method, it sorts the array in ascending alphabetical order. As discussed earlier, to sort the array in descending alphabetical order, we use the reverseOrder() method of Collections class as the second parameter of the sort() method.
Let us see an example to sort the string array:
StringArraySort.java
//importing necessary libraries import java.util.Arrays; import java.util.Collections; public class StringArraySort { public static void main(String[] args) { String str_array[] = {"Guava", "Pineapple", "Apple", "Peach", "Grapes", "Banana"}; System.out.printf("Array before sorting: \n%s\n\n", Arrays.toString(str_array)); //sorting array in ascending order Arrays.sort(str_array); System.out.printf("String array sorted in ascending order: \n%s\n\n", Arrays.toString(str_array)); //sorting array in descending order Arrays.sort(str_array, Collections.reverseOrder()); System.out.printf("String array sorted in descending order : \n%s\n\n", Arrays.toString(str_array)); } }
Output:
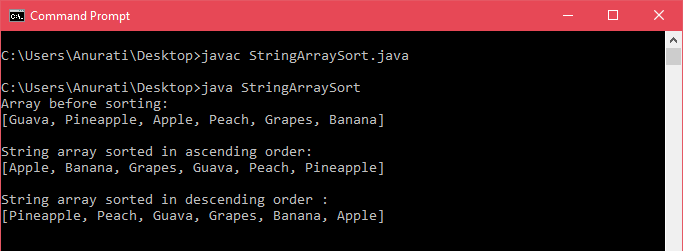
- Sorting a character array
The sort() method also allows the sorting of a char array. There are two types of character array sort:
- Sorting complete array using sort(char[] array_name) method
- Sorting only the specified range of characters using sort(char[] array_name, int fromIndex_var, int toIndex_var) method where fromIndex_var is inclusive and toIndex_var is exclusive.
Let us see how to sort a character array in ascending order using the sort() method.
CharArraySort.java
//importing necessary libraries import java.util.Arrays; public class CharArraySort { public static void main(String[] args) { //Creating a character array char[] charArray = new char[] { 'T', 'F', 'A', 'W', 'B', 'Q', 'M', 'C'}; //Printing the array before sorting System.out.printf("\nChar Array before sorting : %s", Arrays.toString(charArray)); //Sorting the Array Arrays.sort(charArray); //Printing the array after complete sort System.out.printf("\n\nChar Array after sorting : %s", Arrays.toString(charArray)); // Defining another char array char[] charArray2 = new char[] { 'T', 'F', 'A', 'W', 'B', 'Q', 'M', 'C'}; //Sorting a subarray / selective sorting Arrays.sort(charArray2, 2, 8); //Printing selectively sorted array System.out.printf("\n\nChar Array after selective sorting(2,8) : %s \n", Arrays.toString(charArray2)); } }
Output:
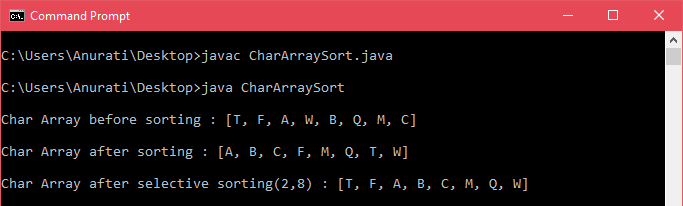
- sort() method of Collections class
The sort() method of Collection class works for the objects Collections like the ArrayList, LinkedList, etc. When the collection elements are of Set type, we can use the TreeSet. To sort the elements of a List type, we use sort() and reverseOrder() methods.
Syntax:
Collections.sort(List list_name)
Consider the following example to understand how to sort the String objects of an ArrayList in ascending order:
CollectionsSort.java
//importing necessary libraries import java.util.*; public class CollectionsSort { public static void main(String[] args) { // Create a list of strings ArrayList<String> array_name = new ArrayList<String>(); array_name.add("Guava"); array_name.add("Pineapple"); array_name.add("Banana"); array_name.add("Apple"); array_name.add("Grapes"); //Printing array before sorting System.out.println("Arrays before sorting:"+ array_name); //to sort in ascending order Collections.sort(array_name); //Printing the sorting array System.out.println("\nArrays after sorting:"+ array_name); } }
Output:

Let’s see an example to sort the String objects in descending order. For this we use reverseOrder() method.
CollectionsSortReverse.java
import java.util.*; public class CollectionsSortReverse{ public static void main(String[] args) { // Create a list of strings ArrayList<String> array_name = new ArrayList<String>(); array_name.add("Guava"); array_name.add("Pineapple"); array_name.add("Banana"); array_name.add("Apple"); array_name.add("Strawberry"); array_name.add("Kiwi"); //Printing array before sorting System.out.println("\nArrays before sorting:"+ array_name); //Sorting array in descending order Collections.sort(array_name, Collections.reverseOrder()); System.out.println("\nDescending array after sorting:"+ array_name); } }
Output:
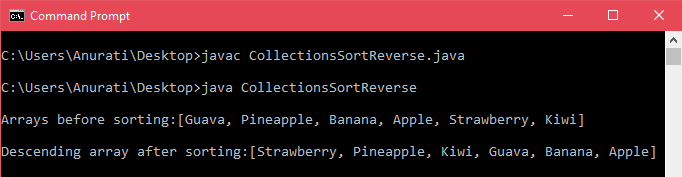
Using the for loop
We can sort an array manually using for loops. The loops traverse the array and compare the adjacent elements and then put them in the proper order.
Here we use two for loops, one to traverse the array from the start and another for loop, which is nested inside the outer loop to traverse the next element.
There are various types of sorting techniques to sort the arrays using for loops.
Click here to learn about different sorting algorithms with examples.
Here we will see the example of the Bubble Sort algorithm, which is the simplest sorting algorithm. In this algorithm, each element of an array is compared to the adjacent element.
ForLoopSort.java
public class ForLoopSort { public static void main(String[] args) { int[] a = {43, 23, 11, 67, 33, 10, 5, 22, 70}; int n = a.length; int i,j; //printing array before sorting System.out.println("\nArray before sorting:"); for(i = 0; i < n; i++){ System.out.print(a[i]+ " "); } for(i=0; i<n; i++) { for (j=0 ;j<n; j++) { if(a[i]<a[j]) { int temp = a[i]; a[i]=a[j]; a[j] = temp; } } } System.out.println("\n\nArray after bubble sort:"); for(i=0; i<n; i++) { System.out.print(a[i]+ " "); } System.out.println(); } }
Output:
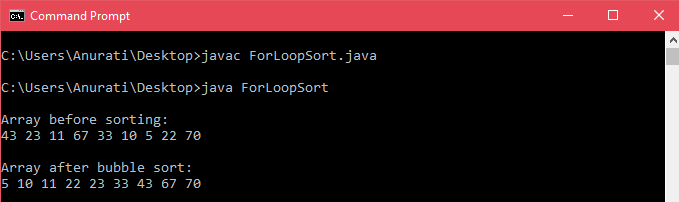
We can also write a user-defined method to sort an array. In the following example, we are using the method named sort_array().
ArraySort.java
public class ArraySort { public static void main(String[] args) { int[] a = {43, 23, 11, -4, 67, 33, 10, -2, 5, 22, 70}; int n = a.length; int i,j; //Printing array before sorting System.out.println("\nArray before sorting:"); for(i = 0; i < n; i++){ System.out.print(a[i]+ " "); } sort_array(a, n); //passing the array and the length of array System.out.println("\n\nArray after bubble sort:"); for(i=0; i<n; i++) { System.out.print(a[i]+ " "); } System.out.println(); } public static void sort_array(int arr[], int len){ for(int i=0; i<len; i++) { for (int j=0 ;j<len; j++) { if(arr[i]<arr[j]) { int temp = arr[i]; arr[i]=arr[j]; arr[j] = temp; } } } } }
Output:
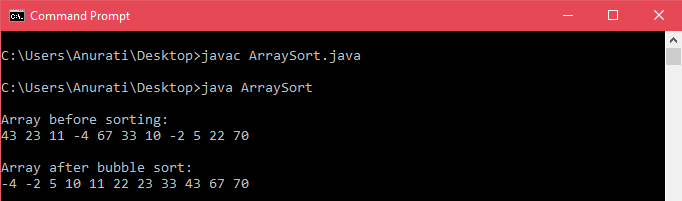
In this way, we have learned how to sort an array in Java using the build-in methods andusing the Java for loop.