Facts about null in Java
Nearly all programming languages have a relationship with null. Hardly any programmers are unconcerned by null. The null has a java.lang.NullPointerException association in Java. Given that it is a class in java.lang package, it is invoked whenever we attempt to carry out specific operations with or without null, and occasionally we aren't even aware of when it has occurred. Every Java programmer needs to be aware of the following significant null-related features in Java:
1. The null is Case Sensitive.
It is not possible to write NULL or 0, as in C, because Java's keywords are case-sensitive. The null is literal in Java.
Filename: Example1.java
public class Example1
{
public static void main (String[] args) throws java.lang.Exception
{
// compile-time error : can't find symbol 'NULL'
Object ob = NULL;
//runs successfully
Object ob1 = null;
}
}
Output
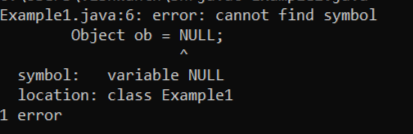
2. Reference Variable value
In Java, the default value of every reference variable is null.
Filename: Example2.java
public class Example2
{
private static Object b;
public static void main(String args[])
{
// it will print null;
System.out.println("Value of object b is : " + b);
}
}
Output

3. Type of null
Contrary to popular belief, the null is not an object or a type. In Simple terms, it's a unique value that can be applied to any reference type, and you can type cast null in any kind.
// null can be assigned to a String
String str = null;
// you can assign null to Integer, also
Integer itr = null;
// Double can also be given a null value.
Double dbl = null;
// null can be type cast to String
String myStr = (String) null;
// it can also be type cast to Integer
Integer myItr = (Integer) null;
// yes, it's possible; no error
Double myDbl = (Double) null;
4. Autoboxing and Unboxing
If a null value is assigned to a primitive boxed data type during auto-boxing and unboxing operations, the compiler throws a Nullpointer exception error.
Filename: Example3.java
public class Example3 {
public static void main(String[] args)
throws java.lang.Exception
{
// This is acceptable because an integer can be null.
Integer i = null;
// Unboxing null to integer throws
// NullpointerException
int a = i;
}
}
Output

5. The instanceof operator
If an object is an instance of the specified type, the Java instanceof operator is used to check this (class, subclass, or interface). If the Expression value is not null, the instanceof operator's result is true at runtime. This is an essential property of instanceof operation, making it useful for type-casting checks.
Filename: Example4.java
public class Example4 {
public static void main(String[] args)
throws java.lang.Exception
{
Integer i = null;
Integer j = 10;
// prints false
System.out.println(i instanceof Integer);
// Compiles successfully
System.out.println(j instanceof Integer);
}
}
Output

6. Static vs Non-static Methods
NullPointerException will be thrown if a non-static method is called on a reference variable with a null value. Still, we can call static methods on reference variables with null values. Static methods won't throw a Null Pointer Exception because they are bound using static binding.
Filename: Example5.java
public class Example5 {
public static void main(String args[])
{
Example5 obj = null;
obj.staticMethod();
obj.nonStaticMethod();
}
private static void staticMethod()
{
// Can be called by null reference
System.out.println(" static method,can be called by null reference & quot");
}
private void nonStaticMethod()
{
// Can not be called by null reference
System.out.print("Non - static method - ");
System.out.println("cannot be called by null reference & quot");
}
}
Output

7. == and !=
In Java, the comparison and not equal operators are permitted with null. This can be used to check for null with Java objects.
Filename: Example6.java
public class Example6 {
public class Example6 {
public static void main(String args[])
{
// return true;
System.out.println(null == null);
// return false;
System.out.println(null != null);
}
}
Output
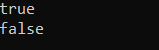
8. The method accepts the argument "null" as a parameter.
In Java, we can print null and pass it as an argument. The argument's data type should be Reference Type. However, depending on the logic of the program, the return type of a method could be any type, such as void, int, double, or any other reference type.
The argument passed from the main method will just be printed in this case by the method "print null."
Program
Filename: Example7.java
import java.io.*;
class Example7 {
public static void print_null(String str)
{
System.out.println("Hey, I am : " + str);
}
public static void main(String[] args)
{
Example7.print_null(null);
}
}
Output

9. ‘+’ operator on null
In Java, the null value can be concatenated with String variables. In Java, it is regarded as a concatenation.
Only the String variable will be concatenated with the null in this case. If the "+" operator is used with null and any other type (such as Double, Integer, etc.) besides String, an error will be raised.
The error message for the integer a=null+7 is “bad operand types for binary operator '+'”.
Program
Filename: Example8.java
import java.io.*;
class Example8 {
public static void print_null(String str)
{
String str1 = null;
String str2 = "_value";
String output= str1+ str2;
System.out.println("Concatenated value : "
+ output);
}
}
Output
