How to create an array in Java
An array is a linear data structure stores a collection of fixed number ofelements of similar type. The size of an array is specified when it is created. The array elements reside in the contiguous memory location.
For example, if we want to store the names of 200 employees, we can create a String type array instead of creating each variable of type String individually.
Some important things about the Java arrays are:
- An array, once created, cannot be modified, i.e., the array size is fixed after the declaration, and the number of elements to be stored is fixed.
- The size of an array must be an int or short value and not along.
- We can declare an array like the other variables just with the square brackets ([]) after the datatype.
- The array elements are stored in sequential order, and each element has an index starting with 0 (a[0], a[1], a[2], …..).
- To find the length of an array in Java, we use the property called length.
For example,
intlen = array_name.length;
- In Java, arrays are treated as an object and are dynamically allocated.
- The Objectclass is the superclass of array type.
- TheCloneableinterface and the Serializable interface are implemented by the array type.
- In Java, an array can contain primitive types like int, char, float, etc., as well as the non-primitive types like an object. For primitive types, values are stored in a contiguous memory location, and for objects, the actual objects are stored in a heap segment.
- Unlike C/C++, Java has the feature of anonymous arrays.
Advantages of an array in Java
- The array elements can be accessed randomly using the array indexes.
- Array elements are simple to retrieve and sort large data sets.
Disadvantages of an array in Java
- Once an array is created, we cannot modify its size. Array size cannot be changed at runtime; therefore, it is called a static data structure.
- An array cannot contain heterogeneous data; it can only contain data of a single primitive type at a time.
Types of arrays
There are two types of arrays in Java:
- One Dimensional array (1-D array):
It is also called a linear array or a single-dimensional array where the elements are stored in a single row.
Example:
intarr[] = new int[10];
- Multidimensional array:
It is the combination of two or more arrays or the nested arrays. In the multidimensional array, more than two rows and columns can be used.
Multidimensional arrays can be two-dimensional (2D arrays) or three-dimensional (3D arrays), we will discuss more about 2D and 3D arrays in this tutorial.
Example:
int[][] arr = new [3][5];
OR
intarr[][] = {{1,2,3}, {4,5,6}, {7,8,9}};
One Dimensional Array in Java

The above diagram showsa 1-D array of size 3. 1-D array or one dimensional array is a linear array where elements are stored sequentially in a row.
The syntax to declare a one-dimensional array is:
data_typearray_name[]; OR data_type []array_name; OR data_type[] array_name;
The data_type is the type of array elements like int, char, float,etc., or non-primitive type like object.
array_name is the name given to an array by the user.
The syntax to instantiate an array in Java:
Instantiating an array means to define the number of elements an array can hold.
array_name = new data_type[size];
Example:
intarr[]; arr = new int[20]; //we can also combine the declaration and instantiation as intarr[] = new int[20];
Here, we have created a one dimensional array of integer type which can storemaximum 20 elements. In other words, size or length of the array is 20.
Note: To get an array is a two-step process. At first, we declare an array of a datatype and a name; then, we allocate the memory to hold the array, using a new operator, and assign it to the array variable we have created. And therefore, all the arrays in Java are dynamically allocated.
Initialization of arrays
In Java, the array elements can be initialized during the declaration.Thus, we can use the given way when the size and array elements are already known.
Example:
int[] roll_no = {101, 102, 105, 111, 130};
Here, roll_no is an array of type int which is initialized with the values given in curly braces.
For initialization, we haven't given the size of the array as the Java compiler itself specifies the size by counting the number of elements in that array. In our case, it is 5.
We don't need to write new int[] in the latest version of Java.
We can also initialize array elements using the indexes as shown below:
//declaring the array int[] roll_no = new int[5]; //initializing the array roll_no[0] = 101; roll_no[1] = 102; roll_no[2] = 105; ..
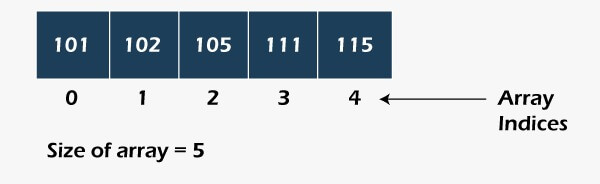
where, array values are stored as
- Array index starts with 0, i.e., the first element of every array is at the index position 0.
- If the array size is n, then the last element of an array is at the index n-1.
Accessing the array elements in Java
Using index numbers
An array can be accessed using its index. As discussed earlier, the index starts with
0 and ends at (size – 1) position.
The syntax to access array element using its index number:
array_name[index];
Let’s consider below example to access array elements using indexes:
ArrayUsingIndex.java
public class ArrayUsingIndex{ public static void main(String[] args) { //declaring and initializing an array int[] roll_no = {101, 103, 105, 100, 111}; //accessing array elements individually using index System.out.println("Printing array elements using index:"); System.out.println("First element: " + roll_no[0]); System.out.println("Second element: " + roll_no[1]); System.out.println("Fifth element: " + roll_no[4]); } }
Output:

In the above example, we access the array elements using its indexes; however, we cannot access each element individually if the array size is large.Here, we can use loops to iterate through the array.
Using the loops
- Accessing an array using for loop:
Commonly, an array is iterated using the for loop in Java. It takes one counter variable (here it is i),which increments until it doesn't reach the end of an array (size – 1).
Syntax:
for(initialization ; condition ; increment/decrement) { //body of the loop }
Consider the following example to understand how to access the array using the fora loop.
ArrayUsingForLoop.java
public class ArrayUsingForLoop{ public static void main(String[] args) { //declaring and initializing an array int[] arr = {11, 34, 21, 77, 20, 2, 44, 78, 100, 66, 12, 30, 7}; //finding the length of array intlen = arr.length; //iterating through the array using for loop System.out.println("\nPrinting array elements using for loop:"); for(inti = 0; i<len; i++) { System.out.print(arr[i] + " "); } System.out.println(); } }
Output:
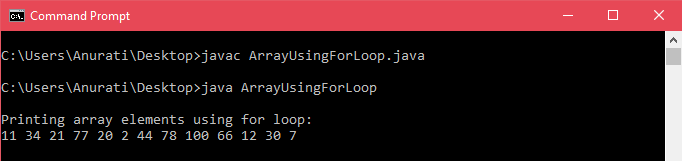
- Accessing an array using for-each loop:
The forEach loop was introduced in Java 5 commonly to iterate over an array or ArrayList. It prints the elements of an array, one by one. At first, it holds the elements in a variable (in our case, the variable is var) and then executes the body of the loop.
Syntax:
for(data_typevariable_name : array_name) { //body of the loop }
Consider following example for iterating array using for-each loop:
ArrayUsingForEach.java
public class ArrayUsingForEach{ public static void main(String[] args) { //declaring and initializing an array int[] arr = {11, 34, 21, 77, 20, 2, 44, 78, 100, 66, 12, 30, 7}; //finding the length of array intlen = arr.length; //iterating through the array using for-each loop System.out.println("\nPrinting array elements using for-each loop:"); for(intvar : arr) { System.out.print(var + " "); } System.out.println(); } }
Output:

Arrays and Methods
- Passing arrays to methods
We can pass arrays to the methods just like we pass variables and values of primitive type. For example, here we are passing an integer array to the method displayArray() as a parameter :
Example:
public static void displayArray(int[] arr) { for(inti = 0 ; i<arr.length ; i++) { System.out.print(arr[i] + " "); } }
We can invoke this method by passing an array while calling it. Consider the example below:
intabc[] = { 2, 4, 22, 78, 21};
displayArray(array_name); // passing the array abc to the method
- Returning array from method
A method can also return the array. Here the return type of the method is the same as that of the array with [].
Let's consider the below example to understand how to return an array from a method. Here, we are returning array rev, which is the reversal of actual array arr.
ReturnArray.java
public class ReturnArray{ //this method returns the reversed array public static int[] reverseArray(int[] arr, int size) { int rev[] = new int[size]; int j = size; for(inti = 0; i< size; i++) { rev[j-1] = arr[i]; j--; } return rev; } public static void main(String[] args) { //creating the array int[] roll = {2,4,6,8,10}; int n = roll.length; inti; System.out.println("Array before reversing"); for(i = 0; i< n; i++){ System.out.print(roll[i] + " "); } //calling the method and passing the array //storing the fetched value in a new array int[] result = reverseArray(roll, n); //printing the fetched values System.out.println("\nArray after reversing"); for(i = 0; i< n; i++){ System.out.print(result[i] + " "); } } }
Output:
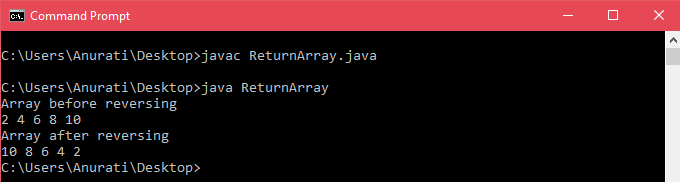
Arrays of Objects
In Java, the array of objects is used to store the objects of a class in an array. The array elements contain the location of reference variables of the object.
Syntax:
class_nameobject_name[] = new class_name[n];
where, n is the number of object references i.e. the array length
Let’s us consider below example to understand the array of objects:
ArrayOfObjects.java
//creating class Student class Student{ introll_no; String name; //constructor of class Student public void Student(int r, String str){ roll_no = r; name = str; } public void displayData(){ System.out.print("Roll no = " +roll_no+ " " + "Name of student = " +name); System.out.println(); } } public class ArrayOfObjects{ public static void main(String[] args) { //creating array of object of class Student Student[] obj = new Student[3]; //creating and initializing the employee objects using constructor obj[0] = new Student(101, "Nirnay"); obj[1] = new Student(102, "Rishi"); obj[2] = new Student(103, "Umang"); //display the Student object data System.out.println("Student object 1:"); obj[0].displayData(); System.out.println("Student object 2:"); obj[1].displayData(); System.out.println("Student object 3:"); obj[2].displayData(); } }
Output:
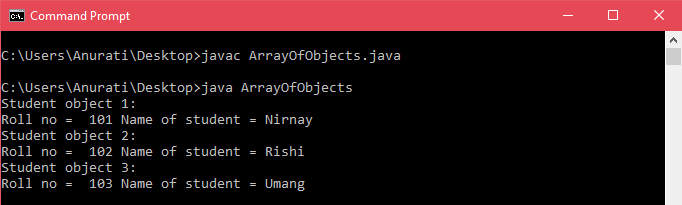
Array exceptions
- ArrayIndexOutOfBoundsException
During the traversal, if the size of an array is negative, or equal to the size of the array or greater than the size of an array, the Java Virtual Machine (JVM) throws ArrayIndexOutOfBoundsException.
It is a runtime exception that is the compiler doesn't check for this error during the compilation.
- NullPointerException
It is also a runtime exception. Following conditions generate the NullPointerException in Java. It is thrown when the application uses null if there is a requirement of an object.
- When the instance method of null object is called
- The field of the null object is accessed or modified.
- Taking the length of null as if it is an array.
- When null is thrown as if it is a Throwable value.
- When the null slots are accessed and modified like an array.
3. NegativeArraySizeException
It is thrown when the array we attempt to create an array of negative length. The NegativeArraySizeException extends the RuntimeException class in Java.
4. IndexOutOfBoundsException
All the indexing pattern data types like array, vector, etc., throw IndexOutOfBoundsexception if they accessed out of their indices (range). This class also extends RuntimeException class.
5. ClassCastException
This exception is thrown when a Java program attempts to cast an object to a subclass that is not its parent class. It is a separate class that extends the class RuntimeException.
6. ArrayStoreException
This exception is thrown when a Java program attempts to store the wrong type of object in the array of objects. It is also a separate class that extends the class RuntimeException.
Example:
Object arr[] = new String[10]; arr[0] = new Double(0);
in the above example, ArrayStoreException is thrown as it tries to store Double object into a String array of objects.
Let’s see the following example to understand the different types of exceptions in array:
ArrayExceptions.java
public class ArrayExceptions{ public static void main(String[] args) { intarr[] = null; int size = 5; intincrementArr = -0; int result; for(inti = 0; i< 7 ; i++){ try{ switch(i){ //gives a NullPointerException case 0: result = arr[0]; break; //gives a NegativeArraySizeException case 1: arr = new int[incrementArr]; result = arr[incrementArr]; break; //gives aArrayIndexOutOfBoundsException case 2: arr = new int[size]; result = arr[size]; break; //gives aIndexOutOfBoundsException case 3: arr = new int[6]; result = arr[6]; //gives a ClassCastException case 4: Object new_arr = new Integer(0); System.out.println((String) new_arr); //gives aArrayStoreException Object obj_arr[] = new String[-5]; obj_arr[0] = new Integer(0); System.out.println(obj_arr); } } catch (NullPointerException exception1){ System.out.println("Exception is a: " +exception1.getMessage()); exception1.printStackTrace(); } catch (NegativeArraySizeException exception2){ System.out.println("Exception is a: " +exception2.getMessage()); exception2.printStackTrace(); } catch (ArrayIndexOutOfBoundsException exception3){ System.out.println("Exception is a: " +exception3.getMessage()); exception3.printStackTrace(); } catch (IndexOutOfBoundsException exception4){ System.out.println("Exception is a: " +exception4.getMessage()); exception4.printStackTrace(); } catch (ClassCastException exception5){ System.out.println("Exception is a: " +exception5.getMessage()); exception5.printStackTrace(); } catch (ArrayStoreException exception6){ System.out.println("Exception is a: " +exception6.getMessage()); exception6.printStackTrace(); } } } }
Output:

Change the array element
We can change the value of a specific element, even if the array is already initialized with the help of an index number.
For example:
city[1] = "Jaipur";
Let’s consider following example. Here we are changing the value of index position 1 from "Delhi" to "Jaipur"
ElementChange.java
public class ElementChange{ public static void main(String[] args) { String city[] = {"Mumbai", "Delhi", "Hyderabad", "Kolkata"}; city[1] = "Jaipur"; //printing the array after element changed for(inti = 0; i<city.length; i++) System.out.println(city[i]); } }
Output:
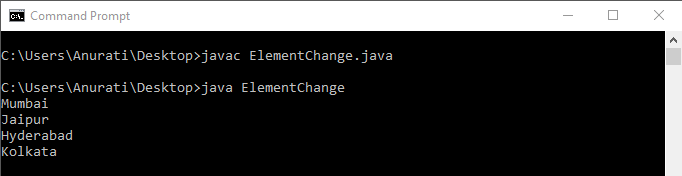
Multidimensional array in Java
It is a type of array which contains one or more arrays. In other words, a multidimensional array can hold multiple rows and columns.
The syntax of a multidimensional array is as follows:
data_type [dimension 1][dimension 2][]...[dimension N] array_name = newdata_type[size 1][size 2]…[size N];
where,
data_type: It is the type of array elements like int, string, float, etc.
dimension: It is the dimension of array like 1D, 2D, etc.
array_name: It is the name of the array.
size: It is the size of the dimension.
Types of Multidimensional Array:
Commonly used multidimensional array in Java is:
- Two-dimensional array (2-D array)
- Three-dimensional array (3-D array)
Calculating the size
To calculate the size of multidimensional arrays, i.e., its total number of elements, we can multiply the size of all dimensions.
- Ifwe declare a 2-D array as:
int[][] arr = new int[5][3];
It will store (5*3) = 15 elements in total.
- And if the array is a 3D array
int[][][] arr = new int[5][7][8];
It will store (5*7*8) = 280 elements.
- Two Dimensional Array:
2D array stores n number of rows and m number of columns. It can be called an array of array (1D array). It is mainly used for games like Super Mario for displaying the screen, displaying spreadsheets, etc.

The syntax to declare a 2-D array is:
data_type[][] array_name = new data_type[r][c];
where, r is the number of rows and c is the number of columns in the array.
Example:
char[][] arr = new char[5][7];
The syntaxto initialize a 2-D array:
array_name[row_index][column_index] = value;
Example:
arr[1][3] = 45;
We can also declare and initialize the array directly as:
data_type[][] array_name = { {valueRow1Column1, valueRow1Column2,…}, {valueRow2Column1, valueRow2Column2,….} };
Example:
int[][] arr = { {11, 12}, {21, 22} };
Accessing the elements of 2D array
The element of a 2D array can be depicted as arr[i][j] where i is the number of rows and j is the number of columns.
Syntax:
arr[row_index][column_index];
If we declare an array as
int[][] arr = new int[2][3];
then we can access its elements directly as
arr[0][1] = 44;
which is the array element located at 1st row and 2nd column.
Let’s consider this example to understand how to create and print the 2-D array. We are creating two matrices and adding them. To display and operate over the elements of two-dimensional array, we use nested for loops i.e., a for loop within another for loop (one is to traverse the rows and another is to traverse the columns).
TwoDimensionalArray.java
public class TwoDimensionalArray{ public static void main(String[] args) { int[][] arr1 = {{1, 2}, {3, 4}}; int[][] arr2 = {{10, 20}, {30, 40}}; int[][] arrSum = new int[2][2]; inti,j; System.out.println("Matrix array 1:"); for(i = 0; i< 2; i++){ for(j = 0; j < 2; j++){ System.out.print(arr1[i][j] + " "); } System.out.println(); } System.out.println("Matrix array 2:"); for(i = 0; i< 2; i++){ for(j = 0; j < 2; j++){ System.out.print(arr2[i][j] + " "); } System.out.println(); } //Adding two matrix arrays for(i = 0; i< 2; i++){ for(j = 0; j < 2; j++){ arrSum[i][j] = arr1[i][j] + arr2[i][j]; } } System.out.println("Sum of matrix array:"); for(i = 0; i< 2; i++){ for(j = 0; j < 2; j++){ System.out.print(arrSum[i][j] + " "); } System.out.println(); } } }
Output:

- Three Dimensional Array

Unlike a 2D array, a 3D array is complex and rarely used in real-time applications. In simpler words, it can be called an array of 2-D array.
The syntax to declare a 3D array is:
data_type[][][] array_name = new data_type[i][r][c];
Example:
int[][][] arr = new int[10][12][15];
The syntax to initialize the 3D array is:
array_name[array_index][row_index][column_index] = value;
Example:
arr[2][3][1] = 34;
We can also declare and initialize 3D array together as:
data_type[][][] array_name = { { { valueArray1Row1Column1, valueArray1Row1Column2,…}, { valueArray1Row2Column1, valueArray1Row2Column2, ….}}, { { valueArray2Row1Column1, valueArray2Row1Column2,…}, { valueArray2Row2Column1, valueArray2Row2Column2, ….}} };
Example:
int[][][] arr = { {{1, 2}, {3, 4}}, {{5, 6}, {7, 8}} };
Accessing the elements of 3D array
The element of a 3D array can be depicted as arr[i][j][k], where i is the number of arrays, j is the number of rows and k is the number of columns.
Syntax:
arr[array_index][row_index][column_index];
If we declare an array as
int[][][]arr = new int[2][3][5];
then we can access its elements directly as
arr[0][1][2] = 44;
which is the element located at second row and third column of the first array of a 3D array.
Consider the following example to understand how to create and display a 3D array. Like the 2D array, we need to use nested loops for displaying and operating over a 3D array. However, we use three for loops in the case of the 3D array, one for traversing the arrays, the second for the rows, and the third for the columns.
ThreeDimensionalArray.java
public class ThreeDimensionalArray{ public static void main(String[] args) { //initializing a 3D array containing 3 arrays //with two rows and 3 columns int[][][] arr = {{{1, 2, 3}, {3, 4, 7}}, {{10, 20, 30}, {50, 60, 70}}, {{100, 200, 500}, {300, 400, 800}}}; inti, j, k; System.out.println("Displaying the 3D array:"); for(i = 0; i< 3; i++){ for(j = 0; j < 2; j++){ for(k = 0; k < 3; k++){ System.out.print(arr[i][j][k] + " "); } System.out.println(); } System.out.println(); } } }
Output:

Jagged Array in Java
It is a special type of multidimensional array in Java, where the number of row elements varies. In other words, a jagged array is a 2D array with an odd number of columns.
Consider the below example to understand how to create a jagged array.
JaggedArray.java
public class JaggedArray{ public static void main(String[] args) { //declaring a 2D array int[][] arr = new int[3][]; //instantiating first row of the array with 4 columns arr[0] = new int [4]; //instantiating second row of the array with 2 columns arr[1] = new int[2]; //instantiating third row of the array with 3 columns arr[2] = new int[3]; int count = 0; //initializing the jagged array for(inti = 0; i<arr.length; i++){ for(int j = 0; j <arr[i].length; j++){ arr[i][j] = count++; } } //printing the array for(inti = 0; i<arr.length; i++){ for(int j = 0; j <arr[i].length; j++){ System.out.print(arr[i][j] + " "); } System.out.println(); } } }
Output:

In this way, we have learned how to create different types of arrays in Java.