New Features of Java 14
Features like Switch expressions and text blocks which are previewed in Java 13 version are standardized in Java 14.
Features in Java 14
- Switch Expression
- Text Blocks
- Records
- Null Pointer Exceptions
- Instance of
- Packaging Tool
- Garbage collectors
1.Switch Expressions
Switch expressions were first released in Java 12 versions. But it was only in preview until Java 14 was released in which it was standardized.
Main.java
import java.util.*;
public class Main{
public static void main(String[] args) {
//Declaring a variable for switch expression
Scanner sc=new Scanner(System.in);
System.out.println("Enter the number");
int n=sc.nextInt();
//Switch expression is declared
switch(n){
//Different Case statements are declared
case 10: System.out.println("Entered number is 10");
break;
case 20: System.out.println("Entered number is 20");
break;
case 30: System.out.println("Entered number is 30");
break;
//Default case statement
default:System.out.println("Number is not in 10, 20 or 30");
}
}
}
By using the switch expression, we can modify the code into easier way
Main.java
public class Main{
public static void main(String[] args) {
//Declaring a variable for switch expression
String n= "Cse";
String result= " ";
//Switch expression is declared
switch(n)
{
//Different Case statements are declared
case "Cse" -> result=" computers";
case "ece" -> result= " electrical";
//Default case statement
default-> result="B.Tech";
}
System.out.println(result);
}
}
2. Text Blocks
Additional two escape characters were added to Java 14 version compared to Java 13 version.
- \: Indicates the end of the line
- \s: Indicates a single space
Previously in Java 13 the text blocks were written as below
Main.java
import java.io.*;
import java.util.*;
public class Main{
public static void main(String[] args)
{
// text is given
String text = "Hello everyone ; Welcome to Java 14 Features";
System.out.println(text);
} // Main
} // class
Now with the new features of Java 14 we can write the above text as
Main.java
import java.io.*;
import java.util.*;
public class Main{
public static void main(String[] args)
{
// text is given
String text =" Hi Everyone \n Welocme";
System.out.println(text);
} // Main
} // class
Output:
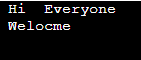
3. Pattern Matching
We use the instance of keyword to check whether the object is an instance of a class or not.
Main.java
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
Main Obj = new Main();
System.out.println(Obj instanceof Main);
// prints true
}
}
4. Records
- Within a record, you can utilize nested classes and interfaces.
- You can also have nested records, which are automatically static.
- Interfaces may be implemented by a record.
- A generic record class can be made.
- Serializing data is possible.
5. Null Pointer Exceptions
When an object reference to a null value, then the NullPointerException is raised.
TestSingleton.java
import java.util.UUID;
import java.io.*;
class Main
{
private static Singleton single = null;
private String ID = null;
Singleton()
{
ID = UUID.randomUUID().toString();
}
public static Singleton getInstance()
{
if (single == null)
single = new Singleton();
return single;
}
public String getID()
{
return this.ID;
}
}
// Driver Code
public class TestSingleton
{
public static void main(String[] args)
{
Singleton s = Singleton.getInstance();
System.out.println(s.getID());
}
}
6. Memory Accesses
The Java 14 provides Java API, allowing Java applications to access external memory that is stored safely and effectively outside the Java heap.