Read the XLSX file in Java
Excel files contain cells; reading an excel file in Java differs from reading a word file. JDK doesn't have a direct API that can read or write Word or Excel documents. We must rely on Apache POI, a third-party library. t has numerous features, is widely used, and receives strong community support.
Apache POI
A Java API called Apache POI can read and write Microsoft Documents in both.xls and.xlsx format. Classes and interfaces are included.
For reading excel files, the Apache POI library offers two implementations:
- Implementation of the HSSF (Horrible Spreadsheet Format) designates an API compatible with Excel 2003 or previous versions.
- Implementation of XSSF (XML Spreadsheet Format): Indicates that an API is compatible with Excel 2007 or later versions.
Class and Interface used in Apache POI used for reading xlsx files
Classes
- XSSFWorkbook is a class that symbolizes an XLSX file.
- An XLSX file's sheet is represented by the class known as XSSFSheet.
- The class XSSFRow stands for a row in an XLSX file's sheet.
- The class XSSFCell represents a cell in a row of an XLSX file.
Interface
- Workbook
It serves as a representation of an Excel workbook. The workbook is an interface that the book and XSSFWorkbook have implemented. This Interface or sheet represents a worksheet in Excel. A workbook's main component, the sheet, represents a grid of cells. Java.lang.The Sheet interface extends Iterable.
- Row
It is a separate interface that depicts a spreadsheet row. Java.lang.The Row interface extends Iterable. The HSSFRow and XSSFRow concrete classes exist.
- Cell
It serves as an interface. Implementing Cell interfaces are HSSFCell and XSSFCell.
Program for reading xlsx file in Java
ReadXLSX.java
// importing all necessary packages
import Java. io. File ;
import java. io . FileInputStream ;
import java . io . IOException ;
import org . apache . poi . hssf . usermodel . HSSFSheet ;
import org . apache . poi . hssf . usermodel . HSSFWorkbook ;
import org . apache . poi . ss . usermodel . Cell ;
import org . apache . poi . ss . usermodel . FormulaEvaluator ;
import org . apache . poi . ss . usermodel . Row ;
// Creating the main class with name ReadXLSX
public class ReadXLSX {
// Main section where execution starts
public static void main ( String [ ] args )
{
// using try block to prevent the stoppage of execution due to errors
try {
// creating object for the class FileInputStream to take the input from user i .e; xlsx file
FileInputStream f1 = new FileInputStream ( new File ( " example.xlsx " ) ) ;
// creating instance for workbook
XSSFWorkbook workbook1 = new XSSFWorkbook ( f1 ) ;
// creating instance for the class XSSFSheet
XSSFSheet st = workbook1 . getSheetAt ( 0 ) ;
// Using Iterator to iterate through the excel sheet
Iterator < Row > r = st . iterator ( ) ;
// Iterating over each row od the excel sheet
while ( r . hasNext ( ) ) {
// creating variable with name row for Row keyword to iterate through the sheet
Row row = r . next ( ) ;
Iterator < Cell > c = row . cellIterator ( ) ;
// Itearator to iterate each column
while ( c . hasNext ( ) ) {
Cell cell = c . next ( ) ;
// using the switch case
switch ( cell . getCellType ( ) )
{
case Cell . CELL_TYPE_NUMERIC :
System . out . print ( cell . getNumericCellValue ( ) + "t" ) ;
break ;
// if the cell contains string value
case Cell . CELL_TYPE_STRING :
System . out . print ( cell . getStringCellValue ( ) + ) ;
break ;
}
}
System . out . println ( " " ) ;
}
// open excel file should be closed
f1 . close ( ) ;
}
// if Exception is raised, it is caught here
// Exception is the main class which contains all the predefined exceptions in it
catch ( Exception e ) {
e . printStackTrace ( ) ;
}
}
}
Output
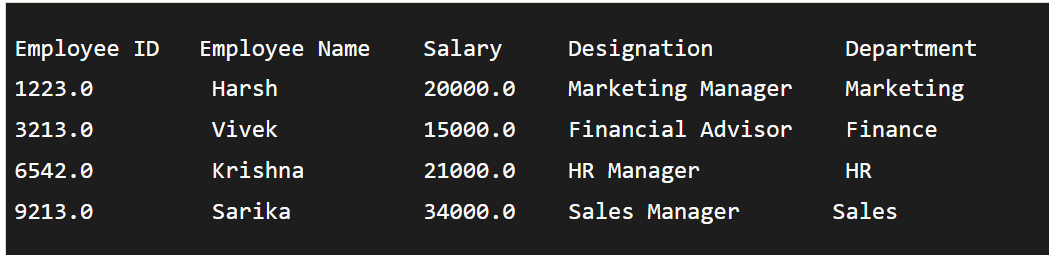