Mutable and Immutable in Java
Java is a programming language in which everything is treated as an object. Its procedures and functions are centred around objects because it is an object-oriented programming language. Mutable and immutable objects are Java object-based concepts, and Java objects are changeable or permanent, depending on how they are iterated.
What are mutable objects?
Objects that can modify their values after initialization are called mutable objects. After that the object is constructed, we can alter its contents for things like fields or states. For instance, StringBuilder, StringBuffer, java.util.Date, etc.
A new item will be generated when we modify a current mutable object; instead, the content of an existing entity will change. The classes for these objects offer ways to modify them.
The concept of mutable objects contains the get() and set() methods.
What are Immutable Objects?
Immutable objects are those whose values cannot be altered once they have been initialized. When an object is formed, it cannot be changed. Primitive objects like int, long, float and double, all legacy classes, the Wrapper class, the String class, etc., are a few examples of immutable objects.
Immutable refers to something that cannot be altered or changed. Immutable objects cannot have their object values or state modified once they have been formed, and immutable objects do not contain the set and get methods.
Creation of a mutable class
For the creation of a mutable class, there are two things are necessary to follow:
- Methods for modification of the field
- Getter and the Setter belonging to the objects
The below example will describe the creation of the mutable class in Java:
Mutable.java
public class Mutable {
private String str;
Mutable(String str) {
this.str = str;
}
public String getName() {
return str;
}
public void setName(String course) {
this.str = course;
}
public static void main(String[] args) {
Mutable ob = new Mutable("Java");
System.out.println(ob.getName());
// By using the setName method name can be updated
ob.setName("Java Programming");
System.out.println(ob.getName());
}
}
Output:

From the example given above, we can change the name of the String by using the setName method.
Creation of immutable class
For the creation of an immutable class, the following things are essential:
- To prevent extensions, a class is designated as final.
- It is recommended that almost all sections be private to restrict access.
- Not a setter
- All mutable fields should be set to final to prevent iteration after initialization.
The below example will describe the creation of an immutable class.
Example 1.
Immutable.java
public class Immutable {
private final String str;
Immutable(final String str) {
this.str = str;
}
public final String getName() {
return str;
}
public static void main(String[] args) {
Immutable object = new Immutable("Java Fundamentals");
System.out.println(object.getName());
}
}
Output:

Example 2
Immutable2.java
// This program is for creating the immutable class
// classes are importing
import java.util.HashMap;
import java.util.Map;
// Class one immutable
final class Teacher {
// Attributes of the final class
private final String str;
confidential last int id;
personal final Map<String, String> data;
// immutable class containing the constructor
//Constructor containing the parameters
public Teacher(String str, int id,
Map<String, String> data)
{
// This keyword is used for reference to the current location
.str = str;
this.id = id;
// By using the HashMap reference is created
// The declaration of the string object
Map<String, String> temporary = new HashMap<>();
// the loop is iterated using the for loop
for (Map.Entry<String, String> entry :
data.entrySet()) {
temporary.put(entry.getKey(), entry.getValue());
}
this.data = temporary;
}
// 1st method
public String getName() { return str; }
// 2nd method
public int getRegNo() { return id; }
// it should not contain any setters
// 3 rd method
// data type of user
public Map<String, String> getMetadata()
{
// By using the HashMap reference is created
Map<String, String> temporary = new HashMap<>();
for (Map.Entry<String, String> entry :
this.data.entrySet()) {
temporary.put(entry.getKey(), entry.getValue());
}
return temporary;
}
}
// Class 2
// Main section
public class Immutable2 {
// Main method
public static void main(String[] args)
{
// With the HashMap as a reference mapping object is created
Map<String, String> mapping= new HashMap<>();
// elements are added to a mapping object
// by using the put() method
mapping.put("1", "one");
mapping.put("2", "two");
Teacher t = new Teacher("Sitara", 1001, mapping);
// The three methods 1,2,3 of class1 are called
// executing the main method of class 2
// and printing the statement
System.out.println(t.getName());
System.out.println(t.getRegNo());
System.out.println(t.getMetadata());
// the incompleteness of the below line in the program
// causes error
// t.id = 102;
mapping.put("3", "three");
// it doesn't change due to the copy of the Constructor
System.out.println(t.getMetadata());
t.getMetadata().put("4", "four");
// it doesn't change
System.out.println(t.getMetadata());
}
}
Output:
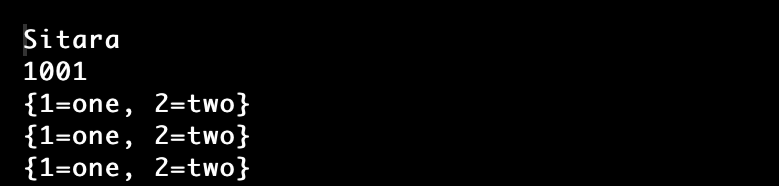
Differences between mutable and immutable objects
The below points will describe the differences between mutable and immutable in Java:
- The variable objects can be updated to any value or state without creating a new thing. In comparison, once an immutable object has been created, it cannot be modified in value or condition. Immutable objects need to create a new thing whenever their state is changed.
- Mutable objects can modify an object's content, and the regular classes do not provide any mechanism to alter the data.
- The mutable objects support the setters and getters. Only setters, no getters, are supported by immutable classes.
- Immutable classes were naturally thread-safe, whereas mutable objects might or might not be thread-safe.
- StringBuffer, Java.util.Date, StringBuilder, etc., were examples of mutable classes. Legacy classes, wrapper classes, the String class, etc., are examples of immutable objects.
The below table will contain the differences:
Mutable | Immutable |
The value for the object can be changed after the initialization. | The value for the object can’t be changed after the initialization. |
The condition is modifiable. | The condition is not adjustable. |
There is no creation of new objects in mutable objects. | Once an object's data is changed, immutable objects create something new. |
It contains ways to modify the object. | It doesn’t contain ways to modify the object. |
It contains both set() and the get() methods. | It contains only the get() method for passing the object. |
Thread safety might or might not apply to mutable classes. | Thread safety will apply to immutable classes. |
Getter and setter methods and strategies for changing fields are necessary for developing mutable classes. | Actual score, private fields, and final mutable objects are necessary for making an immutable class. |
Why are Java Strings immutable?
String in Java is a special kind present in every java program, and strings in Java utilize the fundamental idea. Consider an object with numerous reference variables. In such a case, a reference variable's value would modify the entire object's quality and all of its variables.
Java makes use of the fundamental concept of strings. Think about a reference dynamic array object. In such a scenario, the value of an instance variable would change the quality of the whole thing and all of its variables.
The reason for the immutable nature of the String is:
- If a String in Java is not firm, the String pool cannot exist. JRE reduces the amount of heap space needed. And over one string variable in the collection may refer to the same string variable. If the String were not immutable, String storing would similarly be impossible.
- The String will offer significant dangers to the program if we do not even make this immutable. For instance, database users and passwords are given as strings to get database connections. Additionally provided as strings are the address and line details used for programming. Since the String is immutable, its content cannot be altered. Any hacker might attack the application's security by modifying the reference point if the String were not even kept unchangeable.
- Due to its immutability, the Strings provide security for multithreading. A single "String instance" can be accessed by several threads. Since we explicitly make strings thread-safe, it eliminates the need for synchronization for thread safety.
- Immutability offers the security that the Classloader will load the correct class. Consider a scenario where we attempt to load java.sql. Connection class, but the modifications to the referred value to the hacked. Our database is negatively affected by the Connection class.
Example :
ImmutableString.java
// this program is for string immutability
import java.io.*;
public class ImmutableString {
public static void main(String[] args)
{
String str1 = "online";
str1.concat("courses");
// the string str1 is referring to the string "online."
System.out.println("str1 is referring to " + str1);
}
}
Output

Explanation:
In the above example, string str1 is created containing “ online”. In the second line, the virtual memory stores a new string str1 as “Online courses” but does not refer to anything, so it is lost.