Implicit Typecasting in Java
In this tutorial, we will understand what is meant by implicit typecasting in Java through examples and implementations.
The act of converting one data type into another is known as Typecasting. When the conversion occurs automatically without the programmer's involvement, it is called Implicit Typecasting or Widening Casting. This process involves converting a smaller data type to a larger one, such as converting a byte data type to short, char, int, long, float, or double.
In Java, when two different types of variables are used in an expression, the Java compiler employs a predefined library function to transform the variables into a common data type. The conversion is based on predefined rules.
- If one of the variables is of type double, the other variable's data type will be promoted to double.
- If neither variable is of type double but one is of type float, the other variable's data type will be promoted to float.
- If neither variable is of type double or float but one is of type long, the other variable's data type will be promoted to long.
- If both variables are of type int, they will both be promoted to int.
- If neither variable is of type double, float, long, or int, both variables will be promoted to int.
Let us see the implementation of the above-understood concept.
Implementation:
File name- ImplicitTypecasting1.java
public class ImplicitTypecasting1 {
public static void main(String args[]) {
byte n1 = 30;
System.out.println("byte value : "+n1);
// Implicit Typecasting
short n2 = n1;
System.out.println("short value : "+n2);
int n3 = n2;
System.out.println("int value : "+n3);
long n4 = n3;
System.out.println("long value : "+n4);
float n5 = n4;
System.out.println("float value : "+n5);
double n6 = n5;
System.out.println("double value : "+n6);
}
}
Output:
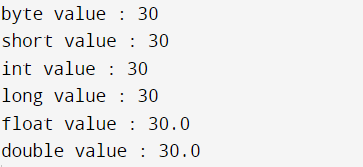
Explanation: The above code is a Java program that demonstrates Implicit Typecasting.
In the code, a byte variable "n1" is declared and initialized with the value of 30. The value of n1 is then printed to the console using the System.out.println() method.
Next, the value of n1 is implicitly typecast to a short variable "n2". The value of n2 is then printed to the console. The process continues, with the value of n2 being implicitly typecast to an int variable "n3", then to a long variable "n4", then to a float variable "n5", and finally to a double variable "n6". The values of n3, n4, n5, and n6 are also printed on the console.
It is to be noted that in each step, the data type is implicitly converted to a larger data type, which is why this process is referred to as Widening Casting.