Non-primitive data types in Java
The kind of data stored in the variable is determined by its type. The type describes the data category (different sizes and values).
These are not already built into the devices. These are created by the user based on the requirements of the user. Non-primitive data types are used for storing different values in a single variable. For example, an array can be used for storing multiple values. Hence these are called the advanced versions of storing values.
The memory address where the data is saved in heap memory, or the storage address where an object is created, is referenced whenever a non-primitive data type is created. A non-primitive data type variable also was known as an object reference variable or a referred data type.
An object reference variable lies on the stack memory, whereas the object it refers to resides in the secondary storage at all times. A reference to the object in a heap is stored in the stack.
All non-primitive data types in Java are referred to as "objects," and they are produced by creating a class.
Key points:
1. For storing any values, it assigns null as default.
2. Anytime we supply a non-primitive data type to a method, we also provide the address of the object containing the data.
Types of Non-primitive data types:
Non-primitive data types are classified into five types in java.
- Class
- Object
- String
- Array
- Interface
Class and Object:
Class is a data type in java created by the user; hence it is called a user-defined data type. It is used as a template for the member variables and methods-based data.
An Object is an instance of the class that can be used for accessing the class elements and methods.
The below example will describe the methods and variables for creating the class. In the example, a class is created which consists of methods( addition( ) and subtraction( ) ). These methods can be accessed from the class by using the object ob.
Datatypes.java
class Datatypes {
// declaring the variables of the class Datatypes
int num1 = 20;
int num2 = 10;
int num3;
// the methods of Datatypes class
public void addition() {
int num3 = num1 + num2;
System.out.println("The sum of the two numbers is: " + num3);
}
public void subtraction() {
int num3 = num1 - num2;
System.out.println("The difference between two numbers: " + num3);
}
// main section
public static void main (String[] args) {
// object ob is created
Datatypes ob = new Datatypes();
//calling the methods of Datatypes class
ob.addition();
ob.subtraction();
}
}
Output:
Interface
Interface and class are similar, but the interface consists of only abstract methods, while a class contains different methods.
Note: If a particular class implements the interface, then all the interface methods should be implemented. Else we can declare it as abstract.
An Interface Calculate is created in the below example, consisting of two abstract methods in the Interface( add( ) and multiply( ) ). The class Example implements the interface, and the interface's methods can be accessed by creating the objects in the Example class.
Example.java
interface Calculate {
void add();
void multiply();
}
public class Example implements Calculate {
// defining the variables of class
int num1 = 10;
int num2 = 20;
int num3;
// the methods of the interface are implemented
public void multiply() {
int num3 = num2 * num1;
System.out.println("The product of two numbers: " + num3);
}
public void add() {
int num3 = num2 + num1;
System.out.println("The sum of two numbers is: " + num3);
}
// main section
public static void main (String[] args) throws IOException {
Example obj = new Example();
// calling the methods using the interface
obj.multiply();
obj.add();
}
}
Output:

String
A string is a group of characters, such as “ Java”, ” Programming”, etc. In java, the string is defined as the class and datatype. Example for string creation in java:
String str= " Java";
Example 1
StringEx.java
public class StringEx {
public static void main(String[] args) {
// a String Str is created and initialised it
String str = "Welcome! An Example of string";
// substring method is used
String subString = str.subString(0,14);
// result of substring
System.out.println(subString);
}
}
Example 2
Palindrome.java
public class Palindrome{
public static void main(String[] args) {
isPalindrome("abc");
isPalindrome("abcba");
isPalindrome("
");
}
private static void isPalindrome(String input) {
boolean res = true;
int len = input.length();
for(int j=0; j < length/2; i++) {
if(input.charAt(i) != input.charAt(length-i-1)) {
res= false;
break;
}
}
System.out.println(input + " is palindrome = "+res);
}
}
Output:

Array
A data type called an array allows for the sequential storage of many homogeneous variables or variables of the same type. They are kept in an indexed format with index zero. The data types of the elements can be either primitive or non-primitive.
Declaring an array with the primitive datatype int:
int age [ ];
The example below describes the initialisation of the array and how to retrieve the array elements using the for a loop.
ArrayEx.java
import java.io. * ;
import java.util. * ;
public class ArrayEx {
public static void main(String[] args) throws IOException {
int i;
Scanner sc = new Scanner(System. in );
// array was declared, and it was initialised
int array[] = {1, 2, 3, 4, 5};
// Another array array1 is declared
int array1[] = new int[5];
// user is requested to enter the values
System.out.println("Put the numbers (size = 5) :");
for (i = 0; i < 5; i++) {
array1[i] = sc.nextInt();
}
System.out.println("Previous array with initialized size is: ");
for (i = 0; i < 5; i++) {
System.out.print(array[i] + " ");
}
System.out.println("\nDynamically the user entered:");
for (i = 0; i < 5; i++) {
System.out.print(array1[i] + " ");
}
}
}
Output:
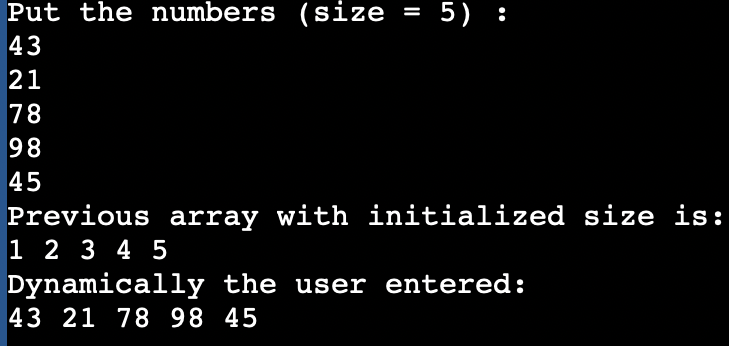