Empty Statement in Java
The three sorts of statements in Java are control, expression, and declaration statements. Additionally, another statement is referred to as an empty statement. In this section, we will discuss about Java's empty statement.
Empty Statement
An empty statement, as its name implies, contains nothing but a semicolon (;). It has numerous applications. Let's talk about some of them.
Empty Statement in a for Loop
for ( int k1 = 0 ; k1 < 10 ; k1++ )
{
// Statements
}
System.out.prinln ( k1 ) ;
As a result, we must refrain from making the variable k's scope local.
int k1 = 0 ;
for( ; k1 < 10 ; k1++ )
{
// statements
}
System.out.prinln ( k1 ) ;
The for loop's initialization block is empty in this case due to the absence of any content.
We can utilize empty statements to build a loop that never ends.
for ( ; ; )
{
// statements
}
The loop can end, nevertheless, if break statements are used inside the loop's body.
An empty statement can be used to construct a for-loop or while loop with no body. Start with the for-loop.
for ( int i1 = 0 ; int i1 < 10 ; i1++ ) ;
The semicolon that follows the for loop indicates that the for loop's body is empty.
The for-loop mentioned above can also be expressed as:
for (int i1 = 0 ; i1 < 10 ; i1++ )
{
// statements
}
Or
for(int i1 = 0 ; i1 < 10 ; i1++)
{
;
}
The for loop body may contain several empty statements.
for(int i = 0 ; i < 10 ; i++)
{
; ; ; ; ; ; ; ; ; ;
}
In this case, the for loop's body contains 10 empty statements.
Empty Statement in while Loop
The while loop can also construct empty statements, just like the for-loop.
int k1 = -1 ;
while ( k1 < 0 ) ;
Since the body of our while loop is an empty expression, it is identical to
int k1 = -1 ;
while ( k1 < 0 )
{
// body
}
The body of the loop can also contain numerous empty statements.
int k = -1 ;
while ( k < 0 )
{
; ; ; ; ; ; ; ; ; ; // 10 empty statements
}
Note that it is meaningless to use several empty statements.
Empty Statement in if … else Statement
int k1 = -1 ;
if ( k1 < 0) ;
The if statement in the code excerpt above has no body since we immediately followed the if statement with a semicolon. We can reformat the code above snippet similarly to loops as:
int k1 = -1 ;
if ( k1 < 0 )
{
}
Similarly, we can immediately follow another statement with a semicolon. Look at the code below.
int k1 = -1 ;
if ( k1 > 0 )
{
// body
}
else ;
We have also taken out the curly braces in this instance for the else part.
Some Common Mistakes
Using empty statements can lead to several frequent errors. Several of them are listed below.
- A compilation error results if we write the else statement right after the if statement and then add two semicolons.
Java Program1 for empty statement
public class EmptyStatement1
{
public static void main(String s[])
{
int k1 = -1;
if ( k1 > 0 ) ; ;
else System . out . println ( " Hi There !! " ) ;
}
}
Output
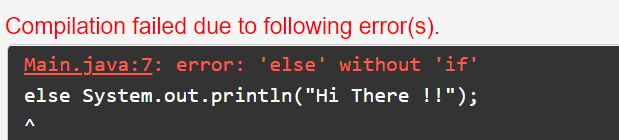
Explanation
This is because we followed the if condition with two semicolons. The first semicolon denotes the if condition's void body and the second semicolon terminates the condition. There is an empty statement between the if part and the else part. It is well known that one cannot insert a statement between the if and else sections, even if it is empty. Therefore, since part one requires curly brackets, the code will generate that compilation error if one attempts to insert two empty sentences.
Empty statement Java Program
public class EmptyStatement2
{
public static void main(String s[])
{
int k1 = -1;
if(k1 > 0)
{
; ;
}
else System.out.println("Hi There !!");
}
}
Output
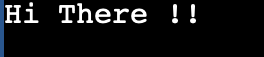
Explanation
The two empty sentences, in this case, make up the if block, followed by the otherwise statement. As a result, the compiler does not object.
Another typical error is to begin the curly braces in the if... else block or the loops following the semicolon.
Empty statement Java Program
public class EmptyStatement3
{
public static void main ( String s [ ] )
{
int k1 = -1 ;
if(k1 > 0) ;
{
System . out . println ( " Hi There !! " ) ;
}
}
}
Output
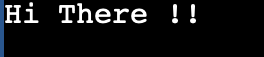
Explanation: The part of the if that follows the curly braces is not part of the if part. The semicolon that follows the if the portion is the reason for this. As a result, even though the print command is enclosed in curly brackets and the predicate (k > 0) is false, Hello is displayed.
Empty statement Java Program
public class EmptyStatement4
{
public static void main ( String s [ ] )
{
for( int j = 0 ; j < 10 ; j++ ) ;
{
System.out.println ( " Hi There !! " ) ;
}
}
}
Output
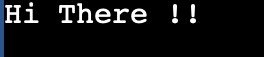
Explanation: After the for-loop, the curly braces are not a part of the for-loop. As a result, the word "Hello" is only printed once.