Inheritance Program in Java
Inheritance Program in Java
Inheritance is one of the important pillars of Object-Oriented Programming that facilitates parent-child relationships in programming. Using inheritance, we can create a new class with the help of an existing class. This newly created class is also known as the derived class, or child class, or subclass, whereas the existing class is known as the base class or parent class, or superclass. The child class acquires the characteristics of the parent class. Inheritance is widely used in the world of programming world as it manages the information in hierarchical order (parent to child, child to sub-child, and so on). The main advantages of using inheritance are:
1) Helps in achieving re-usability of code.
2) Facilitates method overriding in the child class.
Inheritance is mainly used where there is an IS-A relationship. For example,
- Crow is a bird.
- Python is a reptile.
- An employee is a human being.
- Apple is a fruit.
- A Dentist is a doctor.
In the above examples, Crow, Python, Employee, Apple, and Dentist are the child classes, whereas Bird, Reptile, Human Being, Fruit, and Doctor are the parent classes. The Inheritance program in Java shows how the parent-child relationship is achieved via programming.
Syntax:
class XYZ extends ABC { … … }
In Java, the keyword extends is used to achieve inheritance. In the above code snippet, the class XYZ is acquiring the features of the class ABC. Therefore, XYZ is the child class, and ABC is the base class.
Re-usability of code in Inheritance
Consider the following code.
FileName: MainClass.java
class Fruit { String fruitName; } // child class Apple is inheriting parent class Fruit class Apple extends Fruit { } // child class Banana is inheriting parent class Fruit class Banana extends Fruit { } public class Main { public static void main(String argvs[]) { // creating object of the child class Apple Apple apple = new Apple(); // assigning the fruit name apple.fruitName = "Apple"; // displaying the fruit name System.out.println("Fruit name is " + apple.fruitName); // creating object of the child class Banana Banana banana = new Banana(); // assinging the fruit name banana.fruitName = "Banana"; // displaying the fruit name System.out.println("Fruit name is " + banana.fruitName); } }
Output:
Fruit name is Apple Fruit name is Banana
Explanation: In the code, the string field fruitName is declared only in the Fruit class. The two-child classes: Apple and Banana, are inheriting from the Fruit class. Therefore, the string field fruitName is present in the class Apple and Banana. In the driver method, we have created two objects: one each for the class Apple and Banana. Using the created objects, we are accessing the field fruitName for both classes. Thus, we see that whatever we declare in the parent class, the child class automatically gets access to it. We do not have to make the declaration explicitly. This is how the re-usability of code is achieved. Similar to fields, in methods also we can do re-usability of code.
Method Overriding in Inheritance
The method defined in the parent class can get overridden in the child class. The overriding method means changing the definition of the method in the child class. Let’s understand with the help of a program.
FileName: MainClass.java
class Fruit { // declaration of a string field String fruitName; // a method that tells about seeds in a fruit void seeds() { System.out.println("Some fruit has no seeds. "); } } // child class Banana is inheriting the parent class Fruit class Banana extends Fruit { // overriding the method seeds() in the child class Banana @Override void seeds() { System.out.println("Banana has no seeds. "); } } public class MainClass { // driver method public static void main(String argvs[]) { // creating object of the child class Banana Banana banana = new Banana(); // assinging the fruit name banana.fruitName = "Banana"; // displaying the fruit name System.out.println("Fruit name is " + banana.fruitName); // invoking the method seeds() on the object banana banana.seeds(); } }
Output:
Fruit name is Banana Banana has no seeds.
Explanation: The method seeds() is being overridden in the Banana class. Note that, in overriding a method, the definition of the method changes, not it’s signature. Upon invoking the method seeds() on the object banana, the overridden version of the method seeds() is called (observe the output). To stop the overriding, comment/ delete the method seeds() in the child class (do it yourself, and then observe what changes occur in the output.). Before overriding the method, it is a good practice to add “@Override”. This enhances the readability of the code. However, omitting “@Override” does not have any impact on the output.
Note: While overriding a method in Java, we cannot specify a weaker access specifier to an overridden method in the child class. Let’s see what happens if we add the keyword public in the method seeds() of the class Fruit.
FileName: MainClass.java
class Fruit { // declaration of a string field String fruitName; // a method that tells about seeds in a fruit public void seeds() // added keyword public { System.out.println("Some fruit has no seeds. "); } } // child class Banana is inheriting the parent class Fruit class Banana extends Fruit { // overriding the method seeds() in the child class Banana @Override void seeds() { System.out.println("Banana has no seeds. "); } } public class MainClass { // driver method public static void main(String argvs[]) { // creating object of the child class Banana Banana banana = new Banana(); // assinging the fruit name banana.fruitName = "Banana Fruit"; // displaying the fruit name System.out.println("Fruit name is " + banana.fruitName); // invoking the method seeds() on the object banana banana.seeds(); } }
Output: Compilation Error
/MainClass.java:18: error: seeds() in Banana cannot override seeds() in Fruit void seeds() ^
attempting to assign weaker access privileges; was public
1 error
Explanation: The method seeds() has the public access specifier in the parent class, whereas, in the child class, seeds() has the default access specifier, which is weaker than the public access specifier. Thus, we are reducing the visibility of the method seeds(). Hence, we get the compilation error on the execution of the above program.
Types of Inheritance in Java
There are five types of inheritance in Java.
1) Single Inheritance
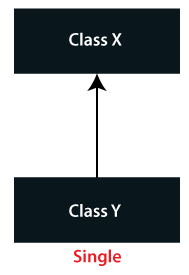
In the above diagram, class Y is acquiring the properties of class X. Therefore, class X is the parent class, and class Y is the child class. The following Java program also demonstrates the same.
// X is the parent class class X { void foo() { System.out.println("In the method foo ... "); } } // Y is the child class class Y extends X { void zoo() { System.out.println("In the method zoo ... "); } } public class SingleInheritance { // driver method public static void main(String argvs[]) { // creating an object of class Y Y y=new Y(); // class Y has herited the method foo() y.foo(); y.zoo(); } }
Output:
In the method foo ... In the method zoo ...
2) Multi-level Inheritance
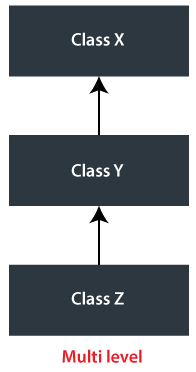
Multi-level inheritance is the extension of the single inheritance. In the above diagram, class Y is acquiring the properties of class X, and class Z is inheriting properties from class Y. Thus, class Y is the child class as it is inheriting properties from class X. Class Y is also the parent class as class Z is acquiring the properties of class Y. Consider the following example.
// X is the parent class class X { void foo() { System.out.println("In the method foo ... "); } } // Y is the child class class Y extends X { void zoo() { System.out.println("In the method zoo ... "); } } // Here, class Y act as the parent class. // class Z is the child class of class Y. class Z extends Y { void coo() { System.out.println("In the method coo ... "); } } public class MultilevelInheritanceExample { // driver method public static void main(String argvs[]) { // creating an object of class Z Z z=new Z(); // class Z has herited the methods foo() and zoo() z.foo(); z.zoo(); z.coo(); } }
Output:
In the method foo ... In the method zoo ... In the method coo ...
3) Hierarchical Inheritance

In hierarchical inheritance, one parent class has more than one child. In the given diagram, class Y and class Z are inheriting properties from class X. The following example illustrates the same.
// X is the parent class class X { void foo() { System.out.println("In the method foo ... "); } void zoo() { System.out.println("In the method zoo ... "); } } // Y is the child class of class X class Y extends X { } // Z is the child class of class X class Z extends X { } public class HierarchicalInheritanceExample { // driver method public static void main(String argvs[]) { // creating an object of class Y Y y=new Y(); // creating an object of class Z Z z=new Z(); // class Y has herited the methods foo() and zoo() y.foo(); y.zoo(); // class Z has herited the methods foo() and zoo() z.foo(); z.zoo(); } }
Output:
In the method foo ... In the method zoo ... In the method foo ... In the method zoo ...
4) Multiple Inheritance
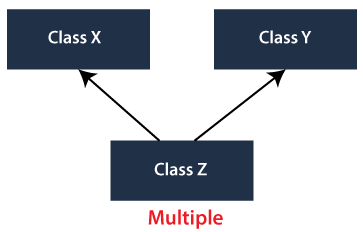
The opposite of multiple inheritance is hierarchical inheritance. In hierarchical inheritance, one child class has more than one parent. Note that multiple inheritance is not supported in Java. To understand the reason behind it, consider the following example.
Filename: MultipleInheritanceExample.Java
// X is the parent class class X { void foo() { System.out.println("In the method foo ... "); } } // Y is also the parent class class Y { void foo() { System.out.println("In the method zoo ... "); } } // Z is the child class of class X and Y class Z extends X, Y { } public class MultipleInheritanceExample { // driver method public static void main(String argvs[]) { // creating an object of class Z Z z=new Z(); // class Z has herited the methods foo() of class X and foo() class Y z.foo(); } }
Output: Compilation Error
Explanation: Class Z is the child class of class X and class Y. Also, the parent classes X and Y contain the method foo() of the same signature. Therefore, the child class Z inherits both the methods foo() mentioned in the classes X and Y. This leads to a dilemma when the method z.foo() is executed because it is not clear whether z.foo() invoking the method foo() of class X or of the class Y. Therefore, to completely eliminate this scenario, Java never supports multiple inheritance.
5) Hybrid Inheritance

The Hybrid inheritance is the mixture of multiple and hierarchical inheritance. The upper part, where class Y and class Z are the children of class X, represents hierarchical inheritance, whereas the lower part, where the class XYZ is acquiring the properties of class Y and class Z, represents multi-level inheritance. Since multi-level inheritance is part of the hybrid inheritance, therefore Java does not support hybrid inheritance.