Java URL Class with Example
Java URL Uniform Resource Locator
To find any resource on the internet, you need to have an address of it.
The URL and IP addresses are the pointers used for this purpose. An IP address points to the physical address of the computer. Instead of all that, the URL contains the protocol to be used, the domain name or an IP address, the path, and optional fragment identifier. It is easy to see that an IP address is the part of a URL, although it is common to see a domain name instead of an IP address.
A domain name specifies the address where Internet users can access your website. As previous computer uses IP addresses, which are a series of numbers, but it is difficult for humans to remember strings of numbers. Because of this, domain names were developed, is the combination of letters and numbers to represent the address to access your website.
URL is an acronym for Uniform Resource Locator. It is used to address resources(document) on the World Wide Web. A resource can be a file or a directory or a more complicated object, like a query to a database or search engine. Web browsers request pages from the web servers by using a URL. A URL refers to a web address.
The Internet is facing a rapid depletion of the IP address pool due to the rapid growth of the internet. IPv6 was invented for increasing the number of IP addresses that can be used over the network. And also, there is no limit to the number of possible URL as there is no shortage of the name.
The syntax of the URL:
Scheme://host:port/path?query-string#fragment-id
The Scheme -It tells about the protocol to be used to access the resource.
The hostname- The hostname indicates where the resources located on the internet.
Port number -It indicates where the server located on the internet.
Path-It specifies the specific resource within the host that the user wants to access.
Query-string-It contains data to be passed on server-side scripts running on the server. It is preceded by a question mark (?), is usually a string of name and value pairs separated by an ampersand.
Fragment-identifier –It is introduced by a hash character (#) is the optional last part of a URL for a document that specifies a location within the page.
How to translate URL:
The web browser finds web pages using an IP or internet protocol. The IP is a series of numbers like 172.217.163.110, and it becomes difficult to remember a number for every website that you want to visit. It is far easier to use a word-based URL like www.google.com. When the host writes a URL into an address field, your web browsers uses DNS(Domain name Server) for translating the URL to the corresponding IP.
It is essential for processing the request through a Domain Name Server(DNS) for converting the domain name into an IP address. Without the DNS, the request would fail as the computer would not be able to find the host. The browser can then use IP numbers to find the information for you.
Syntax:
Creation of the instance of URL: URL url=new URL("https://www.tutorialandexample.com/java-tutorial");
Some constructor for creating URL instances:
Constructor | Description |
URL(String str1) | It creates a URL object from a specified String. |
URL(String protocol, String host11, String file) | It creates a URL object from the specified protocol, host11, and file name. |
URL(String protocol, String host1, int port1, String file1) | It creates a URL object from protocol, host, port, and filename. |
URL(URL url1 ,String str1) | It creates a URL object by parsing the given string in the given context |
URL(String protocol, String host1, int port, String file1, URLStreamHandler handler1) | It creates a URL object from the specified protocol, host, port number, filename, and handler1. |
URL (URL url1, String str, URLStreamHandler handler) | It creates a URL by parsing the given str with the specified handler within a specific context. |
URL provides many methods as follows for parsing the URL:
Method | Description |
public String getProtocol() | It returns the protocol of the URL. |
public String getHost() | It returns the hostname of the URL. |
public String getPort() | It returns the Port Number of the URL |
public String getFile() | It returns the file name of the URL |
public URLConnection openConnection() | It returns the instance of URLConnection. i.e., associated with this URL. |
public String toString() | It returns the String representation of the given URL object |
public String getAuthority() | It returns the authority part of the URL or null if empty. |
public String getPath() | It returns the path of the URL or null if empty. |
public String getQuery() | It returns the query part of the URL. |
public int getDefaultPort() | It returns the default port used. |
Public String getRef() | It returns the reference of the URL |
Types of URL:
- file URL
The file protocol is used in a URL that specifies the location of an operating system file. It is used to retrieve files from within a computer.
Features:
- The file URL is used to designate files accessible on a particular host computer.
- This URL does not designate a resource that is universally accessible over the internet.
Syntax:
file://host/path
Here, the host is the fully qualified domain name of the system on which the path is accessible, and the path is a hierarchical directory path. It specifies the localhost, the machine from which the URL is being interpreted if the host is omitted.
Example-
file:///C:/Users/ABCD/Desktop/socket/1.html
import java.net.*; public class url1{ public static void main(String[] args){ try { URL url=new URL("file:///C:/Users/ABCD/Desktop/socket/1.html"); System.out.println("The Protocol is: "+url.getProtocol()); System.out.println("The Host Name is: "+url.getHost()); System.out.println("The Port Number is: "+url.getPort()); System.out.println("The Default Port Number is: "+url.getDefaultPort()); System.out.println(" The Query String is: "+url.getQuery()); System.out.println("The Path is: "+url.getPath()); System.out.println("File: "+url.getFile()); } catch(Exception e){System.out.println(e);} } }
The output of the code:

2. http URL
The Hypertext Transfer Protocol specifies the application-level protocol for distributed, collaborative, hypermedia information systems. It is the TCP/IP based communication protocol that can be used for delivering the data on the World Wide Web. The data may be the html files, image files, videos, etc. It is a foundation protocol for securing the communication between two systems for e.g., the browsers and the webserver. When you write a URL on your browsers, it sends an http request to the webserver for the webpage. In response, the server sends the http response to the client.
Features:
- The http protocol refers to the request/response protocol working in the client/server- based architecture where the browser acts like http clients, and the server acts as a server. It is a stateless protocol because each command is executed independently without having any knowledge of the commands that came before it.
- It is media independent because any type of data can be sent by it.
- It is connection-less as the server processes the request and sends a response back, after which the client disconnects the connection.
Syntax:
http://host:port/path/file
Example:
http://www.tutorialandexample.com/static-and-dynamic-binding-in-java
import java.net.*; public class url1{ public static void main(String[] args){ try { URL url=new URL("http://www.tutorialandexample.com/static-and-dynamic-binding-in-java"); System.out.println("The Protocol is:"+url.getProtocol()); System.out.println("The Host Name is:"+url.getHost()); System.out.println("The Port Number is:"+url.getPort()); System.out.println("The Default PortNumber is: "+url.getDefaultPort()); System.out.println(" The Query String is:"+url.getQuery()); System.out.println("The Path is:"+url.getPath()); System.out.println("File: "+url.getFile()); } catch(Exception e){System.out.println(e); } } }
The output of the above program:

3. https URL
The https is not a separate protocol from http. It simply uses TLS/SSL encryption over the http protocol. The https prevents the websites from having their information broadcasted in a way that is easily viewed by anyone on the network. With https, traffic encrypts in such a way that even if the packets are intercepted, they will come across as nonsensical characters.
Features:
- The https stands for secure hypertext transfer protocol. It is the secure version of http, by which the data is sent in a secure form between your browsers and the websites to which you are connected.
- It means all communications between your browsers and the website are encrypted.
- The importance of https over the http is the safety of our data from middle hackers as it is encrypted.
- It is mainly for secure web pages, like online banking and online shopping order forms.
Syntax:
https://host:port/path/file
Example:
https://www.tutorialspoint.com/index.htm
import java.net.*; public class url1{ public static void main(String[] args){ try { URL url=new URL("https://www.tutorialspoint.com/index.htm"); System.out.println("The Protocol is: "+url.getProtocol()); System.out.println("The Host Name is: "+url.getHost()); System.out.println("The Port Number is: "+url.getPort()); System.out.println("The Default Port Number is: "+url.getDefaultPort()); System.out.println(" The Query String is: "+url.getQuery()); System.out.println("The Path is: "+url.getPath()); System.out.println("File: "+url.getFile()) ; } catch(Exception e) {System.out.println(e); } } }
The output:

4. ftp URL
ftp is the standard network protocol used for transferring computer files between a client and server on a client-server computer network. It is also used for downloading or uploading the files from or to the remote host.
Features:
- File Transfer protocol URL is the standard mechanism provided by TCP/IP for copying a file from one device to another device.
- It is used for downloading or uploading files between a client and a server on a computer network in a most secure way.
- It allows the back and forth transferring of the files.
Example: To show file Transfer and file Receiver by using the ftp protocol
File Transfer
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.io.*; import java.net.*; public class FileTransfer extends JFrame implements ActionListener { JFrame jf; JButton jb1,jb2; TextField tf; JFileChooser jfc; Socket s; DataInputStream din; DataOutputStream dout,dout1; String s1=new String(); String s2=""; File f; FileTransfer() { jf=new JFrame("File Transfer"); jf.setSize(400,400); Container c=jf.getContentPane(); c.setBackground(Color.red); jf.setLayout(null); jb1=new JButton("choose file"); jb2=new JButton("send"); jb1.setBounds(30,50,100,50); jb2.setBounds(250,150,70,50); jf.add(jb1); jf.add(jb2); tf=new TextField(); tf.setEditable(false); tf.setBackground(Color.white); tf.setBounds(150,50,190,50); jf.add(tf); jf.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); jf.setVisible(true); jfc=new JFileChooser(); jb1.addActionListener(this); jb2.addActionListener(this); } public void fileTransfer(String s1) { try { dout1.writeUTF(s1); dout1.flush(); s2=f.getAbsolutePath(); FileReader fr=new FileReader(s2); BufferedReader br=new BufferedReader(fr); String s3=""; do{ s3=br.readLine(); if(s3!=null) { dout.writeUTF(s3); dout.flush(); } } while(s3!=null); } catch(Exception e) { System.out.println(e+"file not found"); } } public void actionPerformed(ActionEvent e) { if(e.getSource()==jb1) { int x=jfc.showOpenDialog(null); if(x==JFileChooser.APPROVE_OPTION) { f=jfc.getSelectedFile(); String path=f.getPath(); s1=f.getName(); tf.setText(path+"//"+s1); } } if(e.getSource()==jb2) { try { //s1=tf.getText(); s=new Socket("localhost",10); dout1=new DataOutputStream(s.getOutputStream()); dout=new DataOutputStream(s.getOutputStream()); } catch(Exception e1) { System.out.println("send button:"+e1); } fileTransfer(s1); } } public static void main(String a[]) { FileTransfer ft=new FileTransfer(); } }
The output of the program:
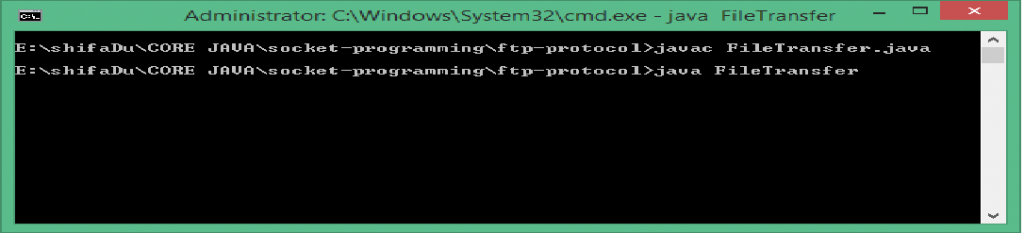
File Receiver
import java.awt.*; import javax.swing.*; import java.io.*; import java.net.*; public class FileReciever { Socket s; ServerSocket ss; DataInputStream dis,dis1; PrintWriter pw; public FileReciever() { try { ss=new ServerSocket(10); s=ss.accept(); System.out.println("connection connected"); dis=new DataInputStream(s.getInputStream()); String s2=dis.readUTF(); System.out.println(s2); FileWriter fr=new FileWriter(s2); pw=new PrintWriter(fr); fileReciever(); } catch(Exception e1) { } } public void fileReciever() { String str=""; try{ do { str=dis.readUTF(); System.out.println(str); pw.println(str); pw.flush(); } while(!str.equals(null)); } catch(Exception e) { } } public static void main(String a[]) { FileReciever fi=new FileReciever(); } }
The output of the program:

Way of addressing the URL:
- Absolute URL? An absolute URL is the complete address of a resource. An absolute URL depends on the protocol, hostname, folder name, and file name. It is similar to the addresses defined by the Indian postal service that contains the name, house number, street address, city, state, and pin code. If any of the information is missing, the mail cannot be delivered to the right person. Similarly, if you miss the information from the URL, the resource file cannot be accessed.
Example 1:
import java.net.*; import java.net.URL; public class absoluteURL { public static void main(String s[]) { try { //It is the complete address of the resource. URL url=new URL("https://www.tutorialandexample.com:80/interface-in-java"); System.out.println(url); //it creates a URL from each component passes as different parameters. URL url1=new URL("https","www.tutorialandexample.com", 80 ,"/java-abstraction"); System.out.println(url1); } catch(MalformedURLException e) { e.printStackTrace(); } } }
The output of the above program:

Example2:
import java.net.*; public class url1{ public static void main(String[] args){ try { URL url=new URL("https://www.google.com/search?q=tutorialandexamplet&oq=tutorialandexample&sourceid=chrome&ie=UTF-8"); System.out.println("The Protocol is: "+url.getProtocol()); System.out.println("The Host Name is: "+url.getHost()); System.out.println("The Port Number is: "+url.getPort()); System.out.println("The Default Port Number is: "+url.getDefaultPort()); System.out.println(" The Query String is: "+url.getQuery()); System.out.println("The Path is: "+url.getPath()); System.out.println("File: "+url.getFile()); } catch(Exception e){System.out.println(e);} } }
Output of program:

- Relative URL –Relative URL does not contain all the parts of a URL, it is always considered with respect to a base URL. A relative URL contains the folder name and file name or only the file name. This URL is basically used when the resource file is stored in the same folder or the same server. In this situation, the browser does not require the protocol and the server name because it assumes the resource file is stored in the folder or the server that is relative to the original file. A relative URL contains enough information to reach the resource relative to another URL. There is a Constructor that creates a URL object from another URL object (the base) and a relative URL specification.
Syntax:
URL(URL baseURL , String relativeURL)
The first argument is the URL object that specifies the base of the new URL. The other argument is a string that specifies rest of the resource name relative to the base. If baseURL is null, then the constructor treats relativeURL like an absoluteURL specification. If relativeURL specifies absolute URL specification, then the constructor ignores baseURL.
Example: We can create a URL that points to https://www.tutorialandexample.com/java-characters/. Then we resolve a URL relative as “../interface-in-java ". The double dots (..) means to go to the parent folder.
import java.net.MalformedURLException; import java.net.URL; public class RelativeURL { public static void main(String s[]) { try { URL base=new URL("https://www.tutorialandexample.com/"); URL rel1=new URL(base," java-characters "); System.out.println("The information of the relative url"); System.out.println(rel1); System.out.println("The Protocol is: "+rel1.getProtocol()); System.out.println("The Host Name is: "+rel1.getHost()); System.out.println("The Port Number is: "+rel1.getPort()); System.out.println("The Default Port Number is: "+rel1.getDefaultPort()); System.out.println(" The Query String is:"+rel1.getQuery()); System.out.println("The Path is:"+rel1.getPath()); System.out.println("File: "+rel1.getFile()); //we create a URL http://www.tutorialandexample.com/java-characters. URL base1=new URL("https://www.tutorialandexample.com/java-characters/"); //we resolve a URL relative as ../ interface-in-java URL rel2=new URL(base1, "../ interface-in-java "); System.out.println("The information of the relative url"); System.out.println(rel2.toExternalForm()); System.out.println("The Protocol is: "+rel2.getProtocol()); System.out.println("The Host Name is: "+rel2.getHost()); System.out.println("The Port Number is: "+rel2.getPort()); System.out.println("The Default Port Number is: "+rel2.getDefaultPort()); System.out.println(" The Query String is: "+rel2.getQuery()); System.out.println("The Path is:"+rel2.getPath()); System.out.println("File: "+rel2.getFile()); } catch(MalformedURLException e) { e.printStackTrace(); }} }
The output of the above program:
