Strontio Number in Java
In this tutorial, we will understandthemeaning of strontio numbers in Java through examples and implementations. These are commonly asked questions in Java interviews.
Strontio Number:
Strontio numbers are those four digits numbers when multiplied by 2 give the same digit at the hundreds and tens place. Remember that the input number must be a four-digit number.
When a four-digit integer is multiplied by 2, the same digit is yielded at the hundredth and tenth place, such a number is known as Strontio Number.
It is to be noted that the considered integer must be of four digits.
Examples:
Let us now see certain examples to get a clearer picture of the topic.
- Let the four-digit number be 666
On multiplying it by 2, we get 6666 * 2 = 13,332
The digit tens place, as well as hundreds place, is 3.
Thus, it is a Strontio number. - Let the four-digit number be 888
On multiplying it with 2, we get 8888 * 2 = 17,776
The digit tens place a, as well as hundreds place, is 7.
Thus, it is a Strontio number. - Let the four-digit number be 331
On multiplying it by, we get 1331 * 2 = 2662
The digit tens place a, as well as hundreds place, is 6.
Thus, it is a Strontio number. - Let the four-digit number be 482
On multiplying it by, we get 1482 * 2 = 2964
The digit at the tens place is 6 and the digit at the hundreds place is 9.
Thus, it is not a Strontio number. - Let the four-digit number be 625
On multiplying it by 2, we get 6625 * 2 = 13250
The digit at the tens place is 5 and the digit at the hundreds place is 2.
Thus, it is not a Strontio number.
Implementation 1: Through Static Input Value
In this approach, a variable with a static input value is declared, and further by using the algorithm one can check if the number is a Strontio number or not.
File name: Strontio_no.java
public class Strontio_no {
public static void main(String args[]) {
//declaring variables to store two different numbers through the static input method
int n1 = 1472;
int n2 = 8888;
//declaring temporary variables to store the input values
int temp1 = n1;
int temp2 = n2;
//removal of all the digits except digits at hundreds and tens place
n1 = (n1 * 2 % 1000) / 10;
n2 = (n2 * 2 % 1000) / 10;
//comparing place value of hundreds with tens
if(n1 % 10 == n1 / 10)
//If the condition is true, the following statement gets printed
System.out.println(temp1+ " is a strontio number.");
else
//This statement gets printed if the condition is false
System.out.println(temp1+ " is not a strontio number.");
if(n2 % 10 == n2/10)
//If the condition is true, the following statement gets printed
System.out.println(temp2+ " is a strontio number.");
else
//This statement gets printed if the condition is false
System.out.println(temp2+ " is not a strontio number.");
}
}
Output:

Implementation 2: Through Input from the user
In this approach, a four-digit number is taken as input from the user, and further, by using this algorithm, one can check if the number is a Strontio number or not.
File name: Strontio_no.java
import java.util.*;
public class Strontio_no {
public static void main(String args[]) {
//creation of an object of Scanner Class
Scanner sc = new Scanner(System.in);
//Taking a four-digit number as input from the user store the no. in a variable
System.out.print("Enter a four digit number: ");
int n1 = sc.nextInt();
//Taking a four-digit number as input from the user store the no. in a variable
System.out.print("Enter a four digit number: ");
int n2 = sc.nextInt();
//declaring temporary variables to store the input values
int temp1 = n1;
int temp2 = n2;
//removal of all the digits except digits at hundreds and tens place
n1 = (n1 * 2 % 1000)/10;
n2 = (n2 * 2 % 1000)/10;
//comparing place value of hundreds with tens
if(n1 % 10 == n1 / 10)
//If the condition is true, the following statement gets printed
System.out.println(temp1+ " is a strontio number.");
else
//This statement gets printed if the condition is false
System.out.println(temp1+ " is not a strontio number.");
if(n2 % 10 == n2 / 10)
//If the condition is true, the following statement gets printed
System.out.println(temp2+ " is a strontio number.");
else
//This statement gets printed if the condition is false
System.out.println(temp2+ " is not a strontio number.");
}
}
Output:
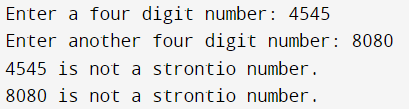
Implementation 3: Through User-defined method
In this approach, a four-digit number is taken as static input and this number is passed as a parameter in a user-defined method, further inside the method, one can check if the number is a Strontio number or not.
File name: Strontio_no.java
import java.util.*;
public class Strontio_no {
//main method
public static void main(String args[]) {
//store a number in a variable by static input method
int n1 = 4000;
//invoke the user-defined method to check if it is a strontio number
if(isStrontio(n1))
//This statement is displayed if the condition is truee
System.out.println(n1+ " is a strontio number.");
else
//The statement is displayed if the condition is false
System.out.println(n1+ " is not a strontio number.");
//declare a variable and store a number to it
int n2 = 6767;
//invoke the user-defined method to check the strontio number
if(isStrontio(n2))
//The statement is displayed if the condition is false
System.out.println(n2+ " is a strontio number.");
else
//The statement is displayed if the condition is false
System.out.println(n2+ " is not a strontio number.");
}
//user-defined method to check if the declared number is a strontio number or not
static boolean isStrontio(int n) {
//declaration of a temporary variable to store the input value
int tmp = n;
//removal of all the digits except hundreds and tens place digit
n = (n * 2 % 1000) / 10;
// comparison of the place value of hundred with tens
if(n % 10 == n / 10)
return true;
else
return false;
}
}
Output:
