Dictionary in Java
Dictionary in Java
The Dictionary class represents a key-value relation which maps keys to values. In Dictionary class, every key and every value is an object.
It is an abstract class associated with Java since JDK 1.0. In Dictionary, every key is associated with at most one value and any object that contains some value can be used as a key and as a value.
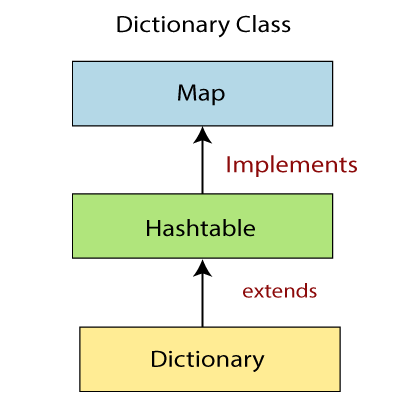
The Dictionary class is deprecated, so new implementation should implement the Map interface, rather than extending this class.
Constructor for Dictionary class:
public Dictionary()
Methods of Dictionary Class
Modifiers | Methods | Description |
abstract v | get(Object key) | This method returns the associated value for the specified key in the argument. Otherwise, returns null. |
put(K key, V value) | It maps the specified key to the specified value in the argument. | |
remove(Object key) | This removes the specified key and its corresponding value from the dictionary. It will do nothing if the specified key is not available in the dictionary. | |
abstract boolean | isEmpty() | It tests whether the dictionary maps keys to the value or not. The result will be true if and only if the dictionary is blank. |
abstract Emumeration<V> | elements() | This method returns an enumeration that will generate all the values contained in the entries of the Dictionary. |
keys() | This method returns a list of the keys present in the dictionary. | |
abstract int | size() | It returns the number of entries (key-value) in the Dictionary. |
Example to illustrate all the above methods:
import Java.util.*; class DictionaryDemo { public static void main(String[] args) { //creating a Dictionary Dictionary d = new Hashtable(); // put() method to insert values along with the key d.put("100", "Tutorials"); d.put("101", "Examples"); // elements() method for emumeration of values: System.out.println( "Elements in Dictionary are : "); for (Enumeration i = d.elements(); i.hasMoreElements();) { System.out.println(i.nextElement()); } // get() method to fetch values from the dictionary : System.out.println("\nValue at key = 50 : " + d.get("50")); System.out.println("Value at key = 100 : " + d.get("100")); // isEmpty() method to check if the dictionary is empty System.out.println("\nThere is no key-value pair : " + d.isEmpty() + "\n"); // keys() method to get all the keys from the dictionary for (Enumeration k = d.keys(); k.hasMoreElements();) { System.out.println("Keys in Dictionary are: " + k.nextElement()); } // remove() method to remove the element at 100 key position System.out.println("\nRemove : " + d.remove("100")); System.out.println("Checking the value of the removed key : " + d.get("100")); System.out.println("\nSize of Dictionary is : " + d.size()); } }
Output:
Elements in Dictionary are : Examples Tutorials Value at key = 50 : null Value at key = 100 : Tutorials There is no key-value pair : false Keys in Dictionary are: 101 Keys in Dictionary are: 100 Remove : Tutorials Checking the value of the removed key : null Size of Dictionary is : 1